Event-based frequency analysis
This sample implements an event based frequency analysis. The generated signal consists of a noisy sine signal with a frequency of 200 Hz and pure noise, which alternate every two seconds. Buffering of the signal begins when a rising edge is detected in the (generated) input signal. The collected data are then relayed via FB_CMA_Source to the function block FB_CMA_MagnitudeSpectrum.
The source code for the sample is available for download from here:
Event_based_FrequencyAnalysis.zip
Block diagram
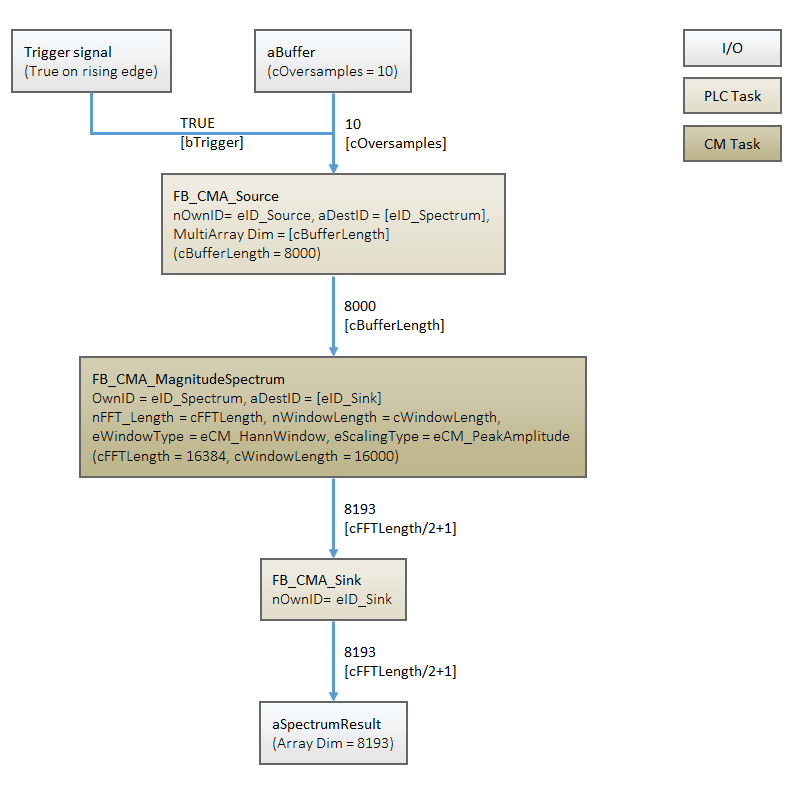
Program parameters
The table below shows a list of important parameters for the configuration of the magnitude spectrum function block.
FFT length | 16384 |
Window size | 16000 |
Buffer size | 8000 |
Window type | eCM_HannWindow |
Scaling type | eCM_ PeakAmplitude |
Event-based buffering of the input signal
The program block CollectData
controls the event-based sampling of the input signal. The input parameters are defined as follows:
PROGRAM CollectData
VAR_INPUT
bTrigger : BOOL; // Trigger signal, start with rising edge
END_VAR
VAR_IN_OUT
aInputSignal : ARRAY[1..cOversamples] OF LREAL; // input time signal
END_VAR
The inverse of the trigger signal bTrigger_
and the current state of buffer are stored locally.
VAR
bTrigger_ : BOOL := FALSE;
nSourceState : UINT := 0;
nActualBuffersSent : ULINT := 0;
nBuffersToSent : ULINT := 2;
// ...
END_VAR
Event-controlled sampling of the signal takes place when the trigger signal has a rising edge and the buffer is ready, i.e. state 0
.
IF (bTrigger AND NOT bTrigger_) AND nSourceState = 0 THEN
nActualBuffersSent := fbSource.nCntResults; // check number of sent MultiArrays from fbSource
fbSourceState := 1;
END_IF
bTrigger_ := bTrigger;
The following code shows the actual event-based buffering of the signal via the source function block.
CASE nSourceState OF
1: // if <nBuffersToSent> MultiArrays has been sent, stop buffering
fbSource.Input1D( pDataIn := ADR(aInputSignal),
nDataInSize := SIZEOF(aInputSignal),
eElementType := eMA_TypeCode_LREAL,
nWorkDim := 0,
pStartIndex := 0,
nOptionPars := 0);
IF (fbSource.nCntResults-nActualBuffersSent) = nBuffersToSent THEN
nSourceState := 2;
END_IF
2: // reset Source Buffer and wait for next trigger hit
fbSource.ResetData();
nSourceState := 0;
END_CASE;
The buffered signal data is subsequently relayed to the magnitude spectrum function block. The buffered signal is processed in the same way as shown in the Magnitude spectrum: sample.
Requirements
Development environment | Target platform | PLC libraries to include |
---|---|---|
TwinCAT v3.1.4018 | PC or CX (x86, x64) | Tc3_CM, Tc3_CM_Base, Tc3_MultiArray |