Histogram
This sample implements a histogram. The code is divided into two tasks: a control task that collects the input data, e.g. from EL3632, and a so-called CM task that calculates the histogram. The block diagram below shows the analysis chain.
The source code for the sample is available for download from here:
Histogram_Sample.zip
Block diagram
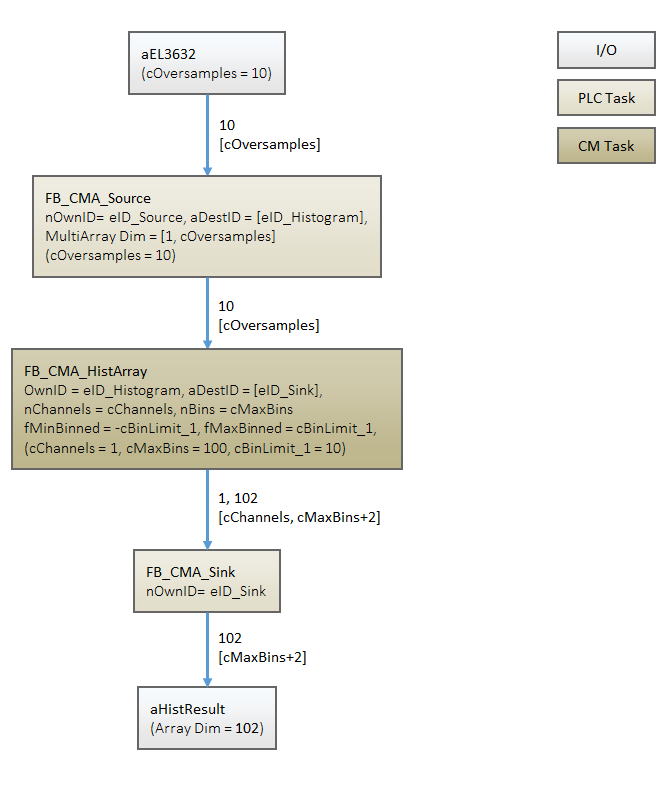
Program parameters
The table below shows the most important parameters for the configuration of the histogram function block:
Histogram Bins |
100 |
Appended Datasets |
10 |
Oversamples |
10 |
Max. Bin Limit |
+3 or +5 |
Min. Bin Limit |
-3 or -5 |
Channels |
1 |
Buffer Length |
100 |
Global Constants
The parameters specified above can be defined as global constants:
VAR_GLOBAL CONSTANT
cBufferLength : UDINT := 100;
cChannels : UDINT := 1;
cOversamples : UDINT := 10;
cMaxBins : UDINT := 100;
cAppendedData : UDINT := 10;
cBinLimit_1 : LREAL := 3;
cBinLimit_2 : LREAL := 5;
END_VAR
Code for the MAIN task
The following code snippet shows the declaration in the MAIN program:
PROGRAM MAIN
VAR CONSTANT
cInitSource : ST_MA_MultiArray_InitPars
:= (eTypeCode := eMA_TypeCode_LREAL, nDims := 2, aDimSizes := [1, cBufferLength]);
END_VAR
VAR
nInputSelection : UDINT := 1;
nSample : UDINT;
aEl3632 AT %I* : ARRAY [1..cOversamples] OF INT;
aBuffer : ARRAY [1..cOversamples] OF LREAL;
fbSource : FB_CMA_Source := (stInitPars := cInitSource, nOwnId
:= eID_Source, aDestIDs := [eID_Histogram]);
fbSink : FB_CMA_Sink := (nOwnID := eID_Sink);
aHistReulst : ARRAY [1..cMaxBins+2];
END_VAR
The following code snippet shows the method calls in the MAIN program:
fbSource.Input2D(pDataIn := ADR(aBuffer),
aDataInSize := SIZEOF(aBuffer),
eElementType := eMA_TypeCode_LREAL,
nWorkDim0 := 0,
nWorkDim1 := 1,
pStartIndex := 0,
nOptionPars := 0);
fbSink(pDataOut := ADR(aHistResult),
nDataOutSize := SIZEOF(aHistResult),
eElementType := eMA_TypeCode_UINT64,
nWorkDim0 := 0,
nWorkDim1 := 1,
nElements := 0,
pStartIndex := 0,
nOptionPars := 0);
Code for the CM task
The variable declaration in the MAIN_CM program:
VAR CONSTANT
cInitHistArray : ST_CM_HistArray_InitPars := (nChannels := cChannels, nBins := cMaxBins, fMinBinnded := -cBinLimit_1, fMaxBinned := cBinLimit_1);
END_VAR
The method calls in the MAIN_CM program:
fbHistArray.CallEx(nAppendData := cAppendData, bReset := );
IF bConfig then
fbHistArray.Configure(pArg := ADR(aHisArrayConfig), nArgSize := SIZEOF(aHistArrayConfig)
END_IF
The Configure method is optional, but it enables the fine setting of the parameters fMinBinned and fMaxBinned at runtime.
Random Number Generator
A histogram is very often used as a visual help in order to understand the underlying distribution of all measured values, e.g. the peaks in the vibration signal. The function generator contained in the sample code is extended for this purpose. The function generator can simulate the usual and practically oriented random numbers and their distributions. Using the variable E_DistributionType you can select a distribution such as exponential, normal (or Gaussian), Chi-squared or gamma. By default the random numbers are generated from a uniform distribution.
![]() | Please note that every distribution requires one or more parameters in order to determine the propagation of the random numbers or their range. This can be done using the input variable aRange. |
Requirements
Development environment | Target platform | PLC libraries to include |
---|---|---|
TwinCAT v3.1.4016.12 | PC or CX (x86, x64) | Tc3_CM (v1.0.19), Tc3_CM_Base, Tc3_MultiArray |