FB_ALY_TimeBasedEnvelope_1Ch
The Time Based Envelope 1Ch algorithm checks whether the periodic input data is within a configured range of values read from a file. This can be a reference signal that was previously learned with Time Based Teach Path 1Ch, for example. The comparison starts when the signal of the Start Period flag is TRUE. It is recommended not to use Time Based Envelope 1Ch simultaneously with Time Based Teach Path 1Ch due to concurrent file access. Instead, a reference signal should first be taught in with Time Based Teach Path 1Ch and only then should the evaluation be carried out with the aid of the Time Based Envelope 1Ch.
Syntax
Definition:
FUNCTION_BLOCK FB_ALY_TimeBasedEnvelope_1Ch
VAR_OUTPUT
ipResultMessage: Tc3_EventLogger.I_TcMessage;
bError: BOOL;
bNewResult: BOOL;
bConfigured: BOOL;
bBusy: BOOL;
eState: E_ALY_ReadState;
bExecutingCompare: BOOL;
nValueNumber: ULINT;
fValueRead: LREAL;
fBandLower: LREAL;
fBandUpper: LREAL;
bWithinBand: BOOL;
eCompareResult: E_ALY_Classification_Bounds;
nCurrentComparedCycles: ULINT;
nCountWithinBand: ULINT;
nCountSmaller: ULINT;
nCountBigger: ULINT;
stFileHeader: ST_ALY_FileHeader;
END_VAR
Outputs
Name | Type | Description |
---|---|---|
ipResultMessage | Contains more detailed information on the current return value. This special interface pointer is internally secured so that it is always valid/assigned. | |
bError | BOOL | This output is |
bNewResult | BOOL | When a new result has been calculated, the output is |
bConfigured | BOOL | Displays |
bBusy | BOOL |
|
eState | Current state of the FB. Necessary due to asynchronous file access. | |
bExecutingCompare | BOOL |
|
nValueNumber | ULINT | Value number of the data point in the file that is currently being compared. |
fValueRead | LREAL | Value of the data point that is read from a file. |
fBandLower | LREAL | Calculated lower limit. |
bWithinBand | BOOL | Calculated upper limit. |
eCompareResult | Class to which the input values belong (WithinBounds/Smaller/Bigger). | |
nCurrentComparedCycles | ULINT | Number of cycles compared. |
nCountWithinBand | ULINT | Counts how often the values were within the band. |
nCountSmaller | ULINT | Counts how often the values were smaller than the band. |
nCountBigger | ULINT | Counts how often the values were larger than the band. |
stFileHeader | ST_ALY_FileHeader | Header information of the file that was read. |
Methods
Name | Definition location | Description |
---|---|---|
Call() | Local | Method for calculating the outputs for a specific configuration. |
Configure() | Local | General configuration of the algorithm with its parameterized conditions. |
GetBusyState() | Local | This method returns the Busy state of the function block. |
Reset() | Local | Resets all internal states or the calculations performed so far. |
SetChannelValue() | Local | Method for passing values to the algorithm. |
SwitchState_Idle() | Local | Initiating the change from state Read to state Idle. See state diagram. |
SwitchState_Read() | Local | Initiating the change from state Idle to state Read. See state diagram. |
UpdateState() | Local | Updating the state after a state change has been initiated and while the target state has not been reached. |
State diagram
Due to the asynchronous file access in real time applications this function block needs a state machine to prepare and finish file access.
At startup the function block is in Idle state. To compare the incoming data with data from file it has to be switched to state Read. Therefore the method SwitchState_Read() has to be called once to set the function block to the PendingRead state. After that the method UpdateState() has to be called until the function block is in state Read. In this state one or multiple comparison cycles can be proceeded. If the function block should not compare additional cycles it can be set to Idle state again. To initiate the state switch the method SwitchState_Idle() has to be called. After that the method UpdateState() has to be called until the function block is in state Idle.
State diagram for the read procedure of the data:
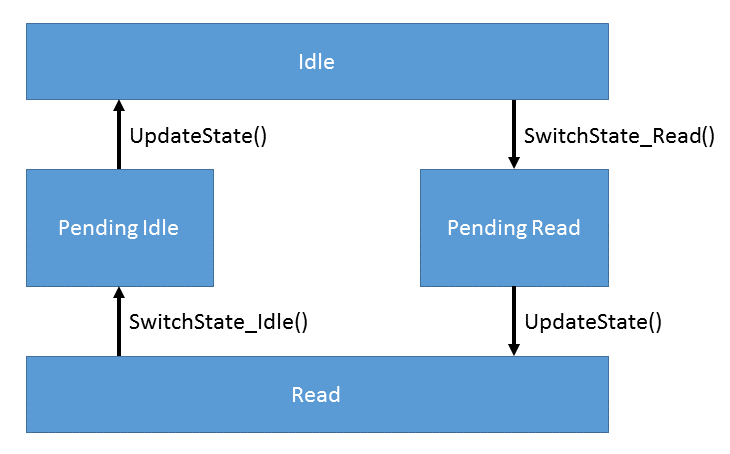
Sample
VAR
fbTimeBasedEnvelope : FB_ALY_TimeBasedEnvelope_1Ch;
eBandMode : E_ALY_BandMode := E_ALY_BandMode.Absolute;
fBand : LREAL := 2.0;
nSegmentSize : UDINT := 200;
tTimeout : TIME := T#5S;
sFilePath : STRING := 'C:\TwinCAT\3.1\Boot\TimeBasedTeach.tas';
bNegateStartPeriod : BOOL := FALSE;
bConfigure : BOOL := TRUE;
eState : E_ALY_ReadState := E_ALY_ReadState.Idle;
bRead : BOOL;
fInput : LREAL;
bStartPeriod : BOOL;
END_VAR
// Configure algorithm
IF bConfigure THEN
bConfigure := FALSE;
fbTimeBasedEnvelope.Configure(eBandMode, fBand, nSegmentSize, tTimeout, sFilePath, bNegateStartPeriod);
END_IF
// Call algorithm
eState := fbTimeBasedEnvelope.eState;
CASE eState OF
E_ALY_ReadState.Idle:
IF bRead THEN
fbTimeBasedEnvelope.SwitchState_Read();
fbTimeBasedEnvelope.UpdateState();
END_IF
E_ALY_ReadState.Read:
fbTimeBasedEnvelope.SetChannelValue(fInput);
fbTimeBasedEnvelope.Call(bStartPeriod:=bStartPeriod);
IF NOT bRead THEN
fbTimeBasedEnvelope.SwitchState_Idle();
fbTimeBasedEnvelope.UpdateState();
END_IF
E_ALY_ReadState.Pending,
E_ALY_ReadState.PendingIdle,
E_ALY_ReadState.PendingRead:
fbTimeBasedEnvelope.UpdateState();
eState := fbTimeBasedEnvelope.eState;
END_CASE
Requirements
Development environment | Target platform | Plc libraries to include |
---|---|---|
TwinCAT v3.1.4024.0 | PC or CX (x64, x86) | Tc3_Analytics |