Export
Exporting the database entries via the server configuration page
In the TcHmiAuditTrail/Audit Trail database tab, the current entries in the database can be exported in JSON or CSV format.
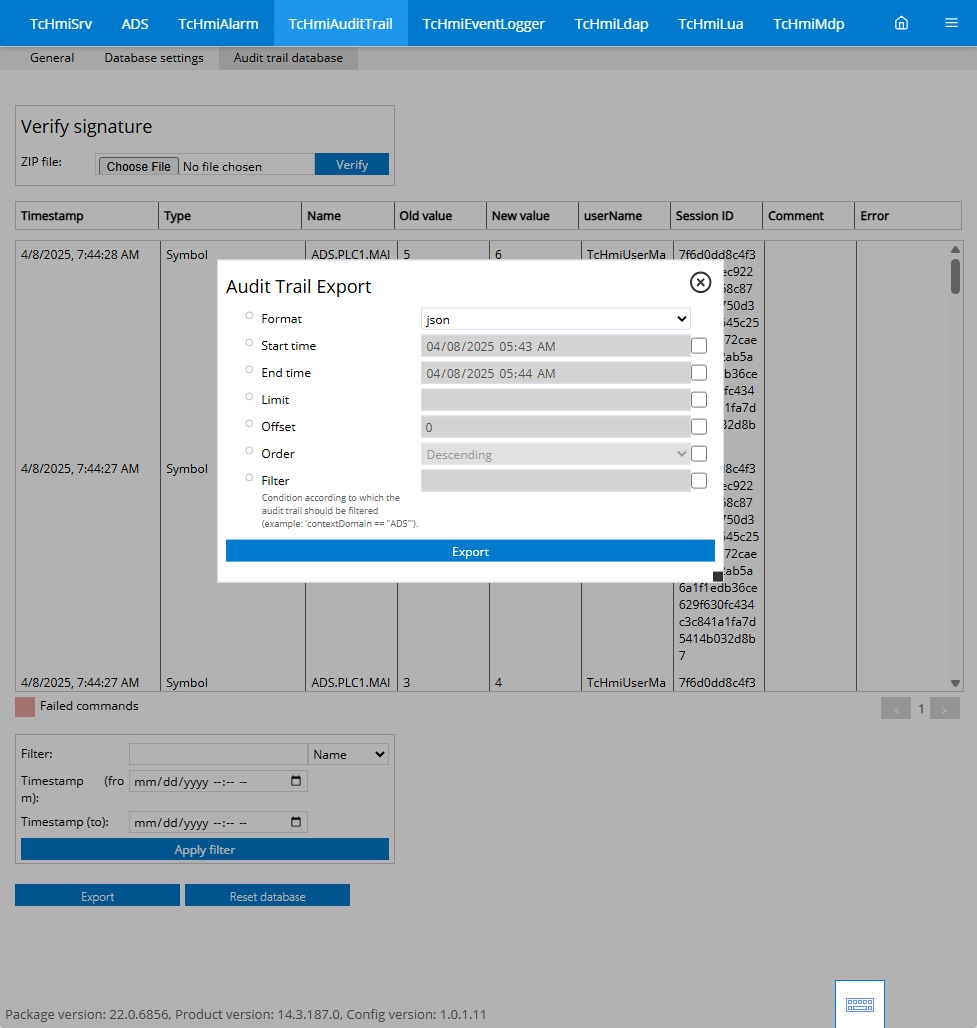
Format
- Selection of the JSON or CSV format to be exported.
Start time
- Start time from which the values are exported.
End time
- Time up to which the data is exported.
Limit
- Limitation of exported entries.
Offset
- Offset from which the data is exported.
Order
- Sort order of the data to be exported.
Filter
- Filter settings for the data to be exported.
Exporting the database entries via the "TcHmiAuditTrailGrid" control
Exporting the database entries via an HTTP request
/**
* Exports and download the audit trails due to the given parameters
*/
// format: 'json' | 'csv',
// limit: number | null,
// offset: number | null,
// orderBy: string | null,
// filter: string | null,
let __exportAuditTrail = function (format, limit, offset, orderBy, filter) {
let url = TcHmi.Environment.getHostBaseUri() + '/Config/ExtensionData/TcHmiAuditTrail';
url += '?format=' + format;
if (filter) {
url += '&filter=' + encodeURIComponent(filter);
}
if (limit) {
url += '&limit=' + limit;
}
if (offset) {
url += '&offset=' + offset;
}
if (orderBy) {
url += '&orderby=' + encodeURIComponent(orderBy);
}
let xhr = new XMLHttpRequest();
xhr.responseType = 'blob';
xhr.addEventListener('load', (_event) => {
if (xhr.status !== 200) {
// handel error
} else {
const blob = new Blob([xhr.response], { type: 'application/zip' });
const url = window.URL.createObjectURL(blob);
const a = document.createElement('a');
a.href = url;
a.download = 'AuditTrail-' + new Date().toISOString().replace(/[:.]/g, '_') + '.zip';
a.click();
window.URL.revokeObjectURL(url);
}
});
const errorHandler = (_event) => {
if (xhr.status === 0) {
// aborted
} else {
// handle error
}
};
xhr.addEventListener('error', errorHandler);
xhr.addEventListener('abort', errorHandler);
xhr.addEventListener('timeout', errorHandler);
xhr.open('GET', tchmi_path(url));
xhr.send();
}