Sample Machine With Microsoft Visual Basic 6.0
TwinCAT ADS OCX
TwinCAT has several programming interfaces in order to integrate applica-tion-specific programs in the system. Visual Basic from Microsoft is one programming language that is sup-ported. The strength of this programming language is the fact that graphi-cal user interfaces are created and it is possible to link them to databases. Visual Basic has been widely spread for some years now and is constantly enhanced by Microsoft. Many third-party vendors offer add-on modules for various areas. The generic term for such modules is OCX.Beckhoff supplies an OCX for TwinCAT that bears the designation Ad-sOCX. AdsOCX provides methods for communicating via the TwinCAT message router with other ADS devices (PLC, NC/CNC, ...).The included example program shows how to access individual variables of the TwinCAT PLC server from Visual Basic.
Access methods:
AdsOCX contains various methods for reading the values out of other ADS devices. The decision as to which method to use depends on the environment in which the program is to be run. You will find further notes and detailed references to the individual functions in the special AdsOCX instructions. Only a brief overview of the individual access methods will be given here:
Access | Meaning |
---|---|
connect | As soon as communication between a PLC variable and a Visual Basic variable is needed, a connection between these two variables is established by activation of a method. During the further course of the program, TwinCAT adapts the Visual Basic variable to the PLC variable. This type of data exchange can also be used so as to activate an event function (event-controlled data transfer) in the event of a change in a PLC variable in the Visual Basic program. |
synchronous | After activation of a read/write method, execution of the Visual Basic program is interrupted until the requested data has arrived. The Program can then continue working with the new data. |
asynchronous | When using asynchronous access, execution of the Visual Basic program is not interrupted and instead, execution of the next command is continued automatically. Once the requested data arrives in AdsOCX, an event function is triggered in the Visual Basic program in which the value is passed on as the parameter |
Sample program
Starting TwinCAT and the PLC program:
TwinCAT and the PLC program must be active before you can start the Visual Basic program.
Starting the Visual Basic program:
Start the 'maschine.exe' program. (TwinCAT\ Samples\First Steps\)
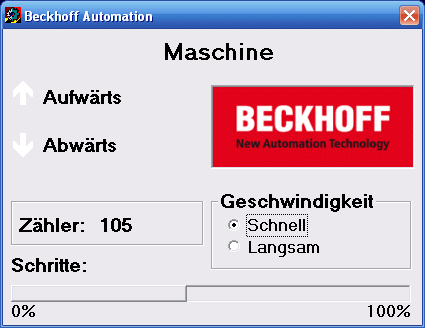
In the left area, you see the two outputs, which are also output to the bus terminals. The variable that counts the workpieces is shown on the bottom left. You can modify the cycle speed of the motor in the 'speed' box. The 'position' display corresponds to the number of cycles that are output to the output 1.
Sourcecode:
Option Explicit
Dim deviceUp As Boolean
Dim deviceDown As Boolean
Dim steps As Integer
Dim counter As Long
Dim hDeviceUp As Long
Dim hDeviceDown As Long
Dim hSteps As Long
Dim hSwitch As Long
Dim hCounter As Long
'-----------------------------------------------------'Is activated first when the program is started'-----------------------------------------------------
Private Sub Form_Load()
'load language dependent words from the resource-file
lblMachine.Caption = LoadResString(0 + GetLanguageId)
lplDeviceUp.Caption = LoadResString(1 +GetLanguageId)
lplDeviceDown.Caption = LoadResString(2 + GetLanguageId)
lblCountLabel.Caption = LoadResString(3 + GetLanguageId)
lplSteps.Caption =LoadResString(4 + GetLanguageId)
fraSpeed.Caption = LoadResString(5 +GetLanguageId)
optSpeedFast.Caption = LoadResString(6 + GetLanguageId)
optSpeedSlow.Caption = LoadResString(7 + GetLanguageId)
'Connect PLC variables with VB variables
Call AdsOcx1.AdsReadIntegerVarConnect(".steps", 2&, 4, 55, steps)
Call AdsOcx1.AdsReadBoolVarConnect(".deviceUp", 2&, 4, 55, deviceUp)
Call AdsOcx1.AdsReadBoolVarConnect(".deviceDown", 2&, 4, 55, deviceDown)
Call AdsOcx1.AdsReadLongVarConnect(".count", 4&, 4, 55, counter)
'Determine handle of the variables
Call AdsOcx1.AdsCreateVarHandle(".steps", hSteps)
Call AdsOcx1.AdsCreateVarHandle(".deviceUp", hDeviceUp)
Call AdsOcx1.AdsCreateVarHandle(".deviceDown", hDeviceDown)
Call AdsOcx1.AdsCreateVarHandle(".count", hCounter)
Call AdsOcx1.AdsCreateVarHandle(".switch", hSwitch)
End Sub
'------------------------------------------'is activated when ending the program'------------------------------------------
Private Sub Form_Unload(Cancel As Integer)
'Separate Visual Basic variables from PLC variables
Call AdsOcx1.AdsReadIntegerDisconnect(steps)
Call AdsOcx1.AdsReadBoolDisconnect(deviceUp)
Call AdsOcx1.AdsReadBoolDisconnect(deviceDown)
Call AdsOcx1.AdsReadLongDisconnect(counter)
'Release handle of the variables
Call AdsOcx1.AdsDeleteVarHandle(hSteps)
Call AdsOcx1.AdsDeleteVarHandle(hDeviceUp)
Call AdsOcx1.AdsDeleteVarHandle(hDeviceDown)
Call AdsOcx1.AdsDeleteVarHandle(hCounter)
Call AdsOcx1.AdsDeleteVarHandle(hSwitch)
End Sub
'------------------------------------------------------'is activated when the 'fast' field is marked'------------------------------------------------------
Private Sub optSpeedFast_Click()
Dim switch As Boolean
'set PLC variable switch to TRUE
switch = True
Call AdsOcx1.AdsSyncWriteBoolVarReq(hSwitch, 2&, switch)
End Sub
'------------------------------------------------------'is activated when the 'slow' field is marked'------------------------------------------------------
Private Sub optSpeedSlow_Click()
Dim switch As Boolean
'set PLC variable switch to FALSE
switch = False
Call AdsOcx1.AdsSyncWriteBoolVarReq(hSwitch, 2&, switch)
End Sub
'------------------------------------------------'is activated when a PLC variable changes'------------------------------------------------
Private Sub AdsOcx1_AdsReadConnectUpdate(ByVal nIndexGroup As Long, ByVal nIndexOffset As Long)
Select Case nIndexOffset
Case hCounter:
'Display quantity in form
lblCount.Caption = counter
Case hDeviceUp
'Adapt colors of graphics according to the variables
DeviceUp_LED.ForeColor = IIf(deviceUp = True, vbRed, vbWhite)
Case hDeviceDown
'Adapt colors of graphics according to the variables
DeviceDown_LED.ForeColor = IIf(deviceDown = True, vbRed, vbWhite)
Case hSteps
'Display position of workpiece
prgSteps.Width = steps * 240
End Select
End Sub
Operating principle:
In Form1_Load() the language dependent text are loaded as resources.The Text will displayed according to the preselected language in Windows NT. The Method ‚AdsReadVarConnect' connects the appropiate VB variables to the PLC System. The parameter of the methods are::
Parameter | Meaning |
---|---|
adsVarName | Name of the PLC variable |
cbLenght | Length of the data in bytes |
nRefreshType | Type of data transfer |
nCycleTime | Refresh cycle in ms |
pData | Visual Basic variable in which the data is written |
The AdsOcx1_AdsReadConnectUpdate() function is activated whenever the variable 'count', 'deviceUp', 'deviceDown' or 'steps' has changed. In it, the objects are animated on the form with the variables of the PLC.
The optFast_Click() and optSlow_Click() functions are activated whenever the user clicks the 'fast' or 'slow' marking box. In these functions, the 'switch' variable is set to TRUE or FALSE. The TwinCAT PLC server reads out this variable and appropriately modifies the behavior of the flashing ('DriveType' function block in the PLC program).
Parameter | Meaning |
---|---|
hVar | Handle of the PLC variable |
cbLenght | Length of the data in bytes |
pData | Visual Basic variable, which contains the data |
When a form is closed, all variables that are no longer needed should be separately from the PLC variables. This is done in the Form1_Unload() function.