Example: machine with Microsoft Visual Basic .NET
Microsoft Visual Studio 2005 is a development environment for creating Visual Basic projects. The machine example is used for familiarisation with the integration of the TwinCAT ADS .NET component with the programming language Visual Basic.
Required software:
- Microsoft .NET Framework Version 2.0, for further information please refer to http://msdn2.microsoft.com
- Microsoft Visual Studio 2005
- TwinCAT 2.10
Before you can start the Visual Basic program, TwinCAT and the PLC program must be active. If Microsoft Visual Studio 2005 is not installed on your computer, please install Microsoft .NET Framework Version 2.0. This sets up the required DLLs on your system.
First steps...
The following steps describe the development of a Visual Basic program and integration of the TwinCAT ADS .NET component.
1. Create new project:
Starting Visual Studio 2005 and creating a new project by selecting -> File -> New -> Project from the menu. A new project based on different templates can be created via the dialog box 'New Project'. Under 'Project Types' select the programming language 'Visual Basic', and under the templates select 'Windows Application'. Enter a new name for your project, in this case please enter 'Machine'. Then select the directory path for your project under 'Location'.
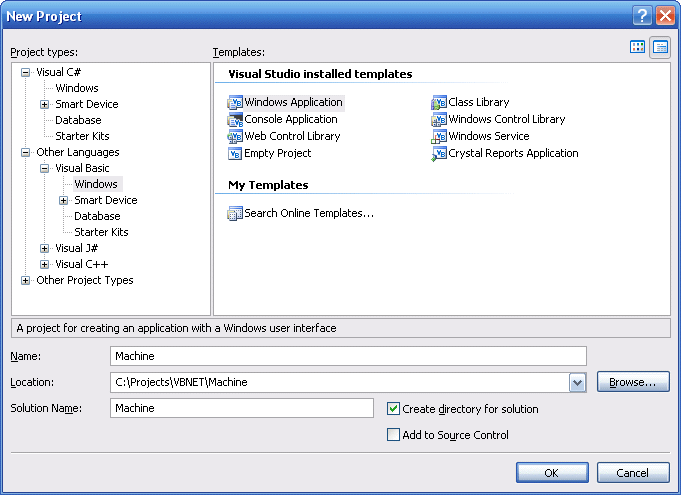
2. Creating a user interface
First create an interface in the design mode of the form 'frmMachine' with the Toolbox. The settings for the different controls (such as 10 labels, 2 radio buttons, 1 progress bar, 1 picture box, group box...) can be viewed and set in the Visual Studio Properties window.
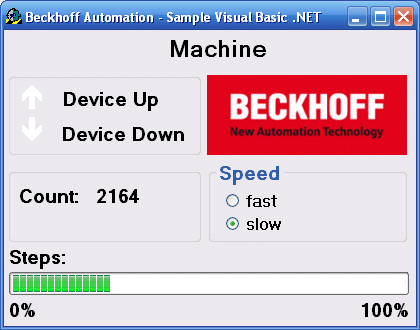
In the upper left you see the two outputs that are also output to the Bus Terminals. The bottom left shows the variable for counting the workpieces. The cycle speed of the motor can be changed via the 'Speed' field on the right. The 'Steps' display shows the number of cycles that are output on output 1.
3. Adding a reference
First a reference called TwinCAT.Ads.dll must be added. The TwinCAT ADS .NET component is integrated via the menu -> Project -> Add Reference.
Further information about integration of the TwinCAT ADS .NET component in Microsoft Visual Studio .NET .
4. Edit source text
Once the interface has been created and the TwinCAT ADS component has been integrated, you can change to the Visual Basic source text. The required namespaces 'System.IO' and 'TwinCAT.Ads' are added in the top row of the source text.
Imports System.IO
Imports TwinCAT.Ads
This is followed by declarations within the frmMachine class.
Private tcClient As TwinCAT.Ads.TcAdsClient
Private dataStream As TwinCAT.Ads.AdsStream
Private binReader As System.IO.BinaryReader
Private hEngine As IntegerPrivate hDeviceUp As IntegerPrivate hDeviceDown As IntegerPrivate hSteps As IntegerPrivate hCount As IntegerPrivate hSwitchNotify As IntegerPrivate hSwitchWrite As Integer
The method 'frmMachine_Load' is started when the 'Windows Application' is called. This method is linked with the method in the Properties window by linking the 'Load' event of the form. It is used to generate instances of different classes and create a link with runtime system 1 of the TwinCAT.Ads component via port 801.
'-----------------------------------------------------
'Wird als erstes beim Starten des Programms aufgerufen
'Is activated first when the program is started
'-----------------------------------------------------
Private Sub frmMachine_Load(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles MyBase.Load
Try
' Create a new instance of the AdsStream class
dataStream = New AdsStream(7)
' Create a new instance of the BinaryReader class
binReader = New BinaryReader(dataStream)
' Create a new instance of the TcAdsClient class
tcClient = New TwinCAT.Ads.TcAdsClient()
' Linking with local PLC - runtime 1 - port 801
tcClient.Connect(801)
Catch ex As Exception
MessageBox.Show("Fehler beim Laden")
End Try
'...
Linking PLC variables:
In the frmMachine_Load event of the form, a connection to each variable in the PLC is created via the TcAdsClient.AddDeviceNotification() method. The handle for this connection is stored in an array. The TransMode parameter specifies the data exchange type. In this case AdsTransMode.OnChange is used to specify that the PLC variable is only transferred if the value in the PLC has changed (see AdsTransMode). The parameter cycleTime determines how often the PLC should check whether the corresponding variable has changed. The variables are then linked with the method 'tcClient_OnNotification' (see "Write method 'tcClient_OnNotifiaction'"), which is called when a variable changes.
Try
' Initialisieren der Überwachung der SPS-Variablen
' Initializing the monitoring of the PLC variables
hEngine = tcClient.AddDeviceNotification(".engine", dataStream, 0, 1, AdsTransMode.OnChange, 10, 0, DBNull.Value)
hDeviceUp = tcClient.AddDeviceNotification(".deviceUp", dataStream, 1, 1, AdsTransMode.OnChange, 10, 0, DBNull.Value)
hDeviceDown = tcClient.AddDeviceNotification(".deviceDown", dataStream, 2, 1, AdsTransMode.OnChange, 10, 0, DBNull.Value)
hSteps = tcClient.AddDeviceNotification(".steps", dataStream, 3, 1, AdsTransMode.OnChange, 10, 0, DBNull.Value)
hCount = tcClient.AddDeviceNotification(".count", dataStream, 4, 2, AdsTransMode.OnChange, 10, 0, DBNull.Value)
hSwitchNotify = tcClient.AddDeviceNotification(".switch", dataStream, 6, 1, AdsTransMode.OnChange, 10, 0, DBNull.Value)
' Holen des Handles von "switch" - wird für das Schreiben des Wertes benötigt
' Getting the handle for "switch" - needed for writing the value
hSwitchWrite = tcClient.CreateVariableHandle(".switch")
' Erstellen eines Events für Änderungen an den SPS-Variablen-Werten
' Creating an event for changes of the PLC variable values
AddHandler tcClient.AdsNotification, AddressOf tcClient_OnNotification
Catch ex As Exception
MessageBox.Show(ex.Message)
End Try
End Sub
The method 'AddDeviceNotification()' was used for linking the variables.
Public Function AddDeviceNotification(ByVal variableName as String, ByVal dataStream as TwinCAT.Ads.AdsStream,
ByVal offset As Integer, ByVal length As Integer, ByVal transMode As TwinCAT.Ads.AdsTransMode,
ByVal cycleTime As Integer, ByVal maxDelay As Integer, ByVal userData As Object)
As Integer
- variableName: Name of the PLC variable.
- dataStream: Data stream receiving the data.
- offset: Interval in the data stream.
- length: Length in the data stream.
- transMode: Event if the variable changes.
- cycletime: Time (in ms) after which the PLC server checks whether the variable has changed.
- maxDelay: Latest time (in ms) after which the event has finished.
- userData: Object that can be used for storing certain data.
The method 'CreateVariableHandle' was used for linking the variable 'hSwitchWrite'.
Public Function CreateVariableHandle(ByVal variableName As String) As Integer
- variableName: Name of the PLC variable.
Write method 'tcClient_OnNotification':
The method is called if one of the PLC variables has changed.
Private Sub tcClient_OnNotification(ByVal sender As Object, ByVal e As AdsNotificationEventArgs)
Try
' Setzen der Position von e.DataStream auf die des aktuellen benötigten Wertes
' Setting the position of e.DataStream to the position of the current needed value
e.DataStream.Position = e.Offset
' Ermittlung welche Variable sich geändert hat
' Detecting which variable has changed
If e.NotificationHandle = hDeviceUp Then
'Die Farben der Grafiken entsprechened der Variablen anpassen
'Adapt colors of graphics according to the variables
If binReader.ReadBoolean() = True Then
DeviceUp_LED.ForeColor = Color.Red
Else
DeviceUp_LED.ForeColor = Color.White
End If
ElseIf e.NotificationHandle = hDeviceDown Then
If binReader.ReadBoolean() = True Then
DeviceDown_LED.ForeColor = Color.Red
Else
DeviceDown_LED.ForeColor = Color.White
End If
ElseIf e.NotificationHandle = hSteps Then
' Einstellen der ProgressBar auf den aktuellen Schritt
' Setting the ProgressBar to the current step
prgSteps.Value = binReader.ReadByte()
ElseIf e.NotificationHandle = hCount Then
' Anzeigen des "count"-Werts
' Displaying the "count" value
lblCount.Text = binReader.ReadUInt16().ToString()
ElseIf e.NotificationHandle = hSwitchNotify Then
' Markieren des korrekten RadioButtons
' Checking the correct RadioButton
If binReader.ReadBoolean() = True Then
optSpeedFast.Checked = True
Else
optSpeedSlow.Checked = True
End If
End If
Catch ex As Exception
MessageBox.Show(ex.Message)
End Try
End Sub
Lastly, we require two methods for making the machine faster or slower. They are used to switch a virtual switch by writing a value to the PLC variable 'switch'.
' Schreiben des Wertes von "switch"
' Writing the value of "switch"
'------------------------------------------------------
'wird aufgerufen, wenn das Feld 'fast' markiert wird
'is activated when the 'fast' field is marked
'------------------------------------------------------
Private Sub optSpeedFast_Click(ByVal sender As Object, ByVal e As EventArgs) Handles optSpeedFast.Click
Try
' Schreiben des Wertes von "switch"
' Writing the value of "switch"
tcClient.WriteAny(hSwitchWrite, True)
Catch ex As Exception
MessageBox.Show(ex.Message)
End Try
End Sub
'------------------------------------------------------
'wird aufgerufen, wenn das Feld 'slow' markiert wird
'is activated when the 'slow' field is marked
'------------------------------------------------------
Private Sub optSpeedSlow_Click(ByVal sender As Object, ByVal e As EventArgs) Handles optSpeedSlow.Click
Try
tcClient.WriteAny(hSwitchWrite, False)
Catch ex As Exception
MessageBox.Show(ex.Message)
End Try
End Sub
To ensure that the methods 'optSpeedFast_Click' and 'optSpeedSlow_Click' are called at the right event, select the radio button 'optSpeedFast' in Design view, click on 'Click' under Events in the Properties window, and select the method 'optSpeedFast_Click'. Proceed accordingly for the radio button 'optSpeedSlow' and the method 'optSpeedSlow_Click'.
Delete notifications and handles:
In the frmMachine_FormClosing event of the form the links are activated again with the method DeleteDeviceNotification().
'------------------------------------------
'wird beim Beenden des Programms aufgerufen
'is activated when ending the program
'------------------------------------------
Private Sub frmMachine_FormClosing(ByVal sender As Object, ByVal e As System.Windows.Forms.FormClosingEventArgs) Handles MyBase.FormClosing
Try
' Löschen der Notifications und Handles
' Deleting of the notifications and handles
tcClient.DeleteDeviceNotification(hEngine)
tcClient.DeleteDeviceNotification(hDeviceUp)
tcClient.DeleteDeviceNotification(hDeviceDown)
tcClient.DeleteDeviceNotification(hSteps)
tcClient.DeleteDeviceNotification(hCount)
tcClient.DeleteDeviceNotification(hSwitchNotify)
tcClient.DeleteVariableHandle(hSwitchWrite)
Catch ex As Exception
MessageBox.Show(ex.Message)
End Try
tcClient.Dispose()
End Sub
You can now start the PLC program Machine_Final.pro in runtime system 1 and then start the VisualBasic program Machine.exe you created.