FB_CTRL_MULTIPLE_PWM_OUT
This block creates PWM modulated output signals from a number of input signals in such a way that the temporal relationships between the output signals are arranged so that as few outputs as possible are switched on at any one time. This temporal arrangement reduces the maximum power necessary required for the actuators.
Both the minimum switch on time and the minimum switch off time can be parameterised, in addition to the mark-to-space ratio, in this extended block.
Description of the output behaviour (1):
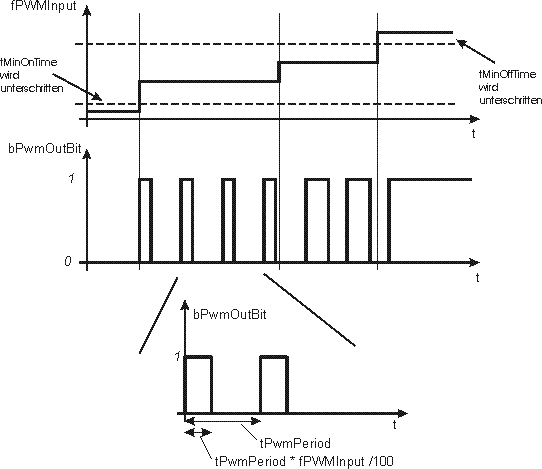
Description of the output behavior (2):
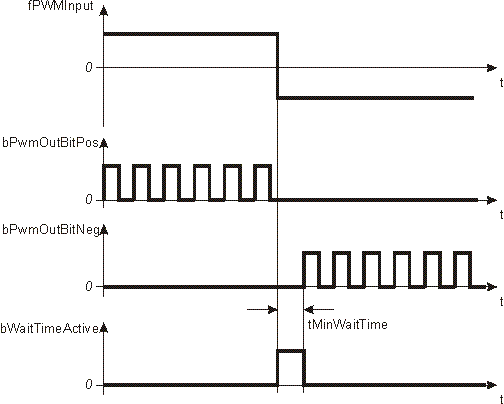
The programmer must create the following arrays in the PLC if this function block is to be used:
ar_fPwmInput :
ARRAY[1..nNumberOfPwmOutputs] OF FLOAT;
ar_stWaitTimesConfig : ARRAY[1..nNumberOfPwmOutputs] OF
ST_CTRL_MULTIPLE_PWM_OUT_TIMES;
ar_stPwmOutputs : ARRAY[1..nNumberOfPwmOutputs] OF
ST_CTRL_MULTIPLE_PWM_OUT_OUTPUTS;
ar_stPwmDataVars : ARRAY[1..nNumberOfPwmOutputs] OF
ST_CTRL_MULTIPLE_PWM_OUT_DATA;
The input values for the individual channels of the PWM function block are written into the ar_fPwmInput array. The programmer can specify the parameterizable times for the individual channels in the ar_stWaitTimesConfig array. The function block writes the output bits into the ar_stPwmOutputs array. The internal data required by the function block is stored in the ar_stPwmDataVars array. Under no circumstances the structures contained in the last-named array may not be changed in the PLC program. This procedure is also illustrated in the sample program listed below.
VAR_INPUT
VAR_INPUT
eMode : E_CTRL_MODE;
END_VAR
eMode : Input that specifies the block's operating mode.
VAR_OUTPUT
VAR_OUTPUT
eState : E_CTRL_STATE;
bError : BOOL;
eErrorId : E_CTRL_ERRORCODES;
END_VAR
eState : State of the function block.
eErrorId : Supplies the error number when the bError output is set.
bError : Becomes TRUE, as soon as an error occurs.
VAR_IN_OUT
VAR_IN_OUT
stParams : ST_CTRL_PWM_OUT_EXT_PARAMS;
END_VAR
stParams : Parameter structure of the PWM element. This consists of the following elements:
TYPE
ST_CTRL_MULTIPLE_PWM_OUT_PARAMS :
STRUCT
tTaskCycleTime : TIME; (* PLC/PWM cycle time in
seconds *)
tPWMPeriod : TIME; (* controller cycle time
in seconds *)
nNumberOfPwmOutputs : USINT;
pPwmInputArray_ADR : POINTER TO FLOAT := 0;
nPwmInputArray_SIZEOF : UINT;
pPwmTimesConfig_ADR : POINTER TO
ST_CTRL_MULTIPLE_PWM_OUT_TIMES;
nPwmTimesConfig_SIZEOF : UINT;
pPwmOutputArray_ADR : POINTER TO
ST_CTRL_MULTIPLE_PWM_OUT_OUTPUTS;
nPwmOutputArray_SIZEOF : UINT;
pPwmData_ADR : POINTER TO
ST_CTRL_MULTIPLE_PWM_OUT_DATA;
nPwmData_SIZEOF : UINT;
END_STRUCT
END_TYPE
tTaskCycleTime : Cycle time with which the function block is called. If the function block is called in every cycle this corresponds to the task cycle time of the calling task.
tPWMPeriod : Period of the PWM signal.
nNumberOfPwmOutputs : Number of PWM outputs. [1...n]
pPwmInputArray_ADR : Address of the PWM input array.
nPwmInputArray_SIZEOF : Size of the PWM input array in bytes.
pPwmTimesConfig_ADR : Address of the PWM times array.
nPwmTimesConfig_SIZEOF : Size of the PWM times array in bytes.
pPwmData_ADR : Address of the internal PWM data array.
pPwmData_SIZEOF : Size of the internal PWM data array in bytes.
TYPE
ST_CTRL_MULTIPLE_PWM_OUT_TIMES :
STRUCT
tMinOnTime : TIME; (* min. switch on time *)
tMinOffTime : TIME; (* min. switch off time *)
tMinWaitTime : TIME; (* min. waiting time when switching from
pos to neg or vv*)
END_STRUCT
END_TYPE
tMinOnTime : Minimum switch-on time of the PWM output.
tMinOffTime : Minimum switch-off time of the PWM output.
tMinWaitTime : Waiting time between switching between a positive and negative output signal.
TYPE
ST_CTRL_MULTIPLE_PWM_OUT_OUTPUTS :
STRUCT
bPwmOutBitPos : BOOL;
bPwmOutBitNeg : BOOL;
bWaitTimeActive : BOOL;
END_STRUCT
END_TYPE
bPwmOutBitPos : PWM signal if fPwmInput > 0.0.
bPwmOutBitNeg : PWM signal if fPwmInput < 0.0.
bWaitTimeActive : A TRUE at this output indicates that the waiting time between the switching actions of the output signals is active. This output can be used to hold an I component that may be present in the prior controller constant during this time.
TYPE
ST_CTRL_MULTIPLE_PWM_OUT_DATA :
STRUCT
Interne Struktur. Auf diese darf nicht schreibend
zugegriffen werden.
END_STRUCT
END_TYPE
Sample:
PROGRAM PRG_MULTIPLE_PWM_OUT_TEST
VAR CONSTANT
nNumberOfPwmOutputs : USINT := 3;
END_VAR
VAR
ar_fPwmInput : ARRAY[1..nNumberOfPwmOutputs] OF
FLOAT;
ar_stWaitTimesConfig : ARRAY[1..nNumberOfPwmOutputs] OF
ST_CTRL_MULTIPLE_PWM_OUT_TIMES;
ar_stPwmOutputs : ARRAY[1..nNumberOfPwmOutputs] OF
ST_CTRL_MULTIPLE_PWM_OUT_OUTPUTS;
ar_stPwmDataVars : ARRAY[1..nNumberOfPwmOutputs] OF
ST_CTRL_MULTIPLE_PWM_OUT_DATA;
eMode : E_CTRL_MODE;
stParams :
ST_CTRL_MULTIPLE_PWM_OUT_PARAMS;
eErrorId : E_CTRL_ERRORCODES;
bError : BOOL;
fbPwm_Out : FB_CTRL_MULTIPLE_PWM_OUT;
bInit : BOOL := TRUE;
fIn1 : FLOAT;
fIn2 : FLOAT;
fIn3 : FLOAT;
bOut1_pos : BOOL;
bOut1_neg : BOOL;
bOut2_pos : BOOL;
bOut2_neg : BOOL;
bOut3_pos : BOOL;
bOut3_neg : BOOL;
END_VAR
IF bInit
THEN
(* set values in the local arrays *)
ar_stWaitTimesConfig[1].tMinOnTime := T#500ms;
ar_stWaitTimesConfig[1].tMinOffTime := T#300ms;
ar_stWaitTimesConfig[1].tMinWaitTime := T#3.5s;
ar_stWaitTimesConfig[2].tMinOnTime := T#400ms;
ar_stWaitTimesConfig[2].tMinOffTime := T#250ms;
ar_stWaitTimesConfig[2].tMinWaitTime := T#4.5s;
ar_stWaitTimesConfig[3].tMinOnTime := T#400ms;
ar_stWaitTimesConfig[3].tMinOffTime := T#200ms;
ar_stWaitTimesConfig[3].tMinWaitTime := T#5.5s;
(* set values in the parameter struct *)
stParams.tTaskCycleTime := T#2ms;
stParams.tPWMPeriod := T#2s;
stParams.nNumberOfPwmOutputs := nNumberOfPwmOutputs;
(* set the ctrl-mode *)
eMode := eCTRL_MODE_ACTIVE;
bInit := FALSE;
END_IF
(* set the addresses *)
stParams.pPwmTimesConfig_ADR := ADR(
ar_stWaitTimesConfig);
stParams.nPwmTimesConfig_SIZEOF := SIZEOF(
ar_stWaitTimesConfig);
stParams.pPwmInputArray_ADR := ADR( ar_fPwmInput );
stParams.nPwmInputArray_SIZEOF := SIZEOF( ar_fPwmInput );
stParams.pPwmOutputArray_ADR := ADR( ar_stPwmOutputs );
stParams.nPwmOutputArray_SIZEOF := SIZEOF( ar_stPwmOutputs );
stParams.pPwmData_ADR := ADR( ar_stPwmDataVars );
stParams.nPwmData_SIZEOF := SIZEOF( ar_stPwmDataVars
);
ar_fPwmInput[1] := fIn1;
ar_fPwmInput[2] := fIn2;
ar_fPwmInput[3] := fIn3;
fbPwm_Out( eMode := eMode,
stParams := stParams,
bError => bError,
eErrorId => eErrorId);
bOut1_pos := ar_stPwmOutputs[1].bPwmOutBitPos;
bOut1_neg := ar_stPwmOutputs[1].bPwmOutBitNeg;
bOut2_pos := ar_stPwmOutputs[2].bPwmOutBitPos;
bOut2_neg := ar_stPwmOutputs[2].bPwmOutBitNeg;
bOut3_pos := ar_stPwmOutputs[3].bPwmOutBitPos;
bOut3_neg := ar_stPwmOutputs[3].bPwmOutBitNeg;