FB_CTRL_PI_PID
The function block provides a cascaded PI-PID controller in the functional diagram. Internally, this block uses the FB_CTRL_PI, FB_CTRL_LIMITER and FB_CTRL_PID transfer elements.
Transfer function of the PI element:
Transfer function of the PID element:
Functional diagram for the cascaded transfer element:
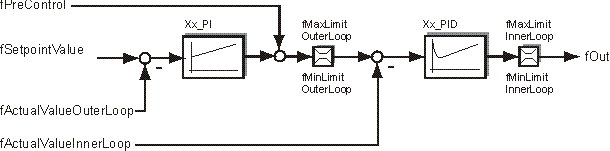
VAR_INPUT
VAR_INPUT
fSetpointValue : FLOAT; (* setpoint value *)
fActualValueOuterLoop : FLOAT; (* actual value from the process to the PI-controller *)
fActualValueInnerLoop : FLOAT; (* actual value from the process to the PID-controller *)
fPreControl : FLOAT; (* pre control value *)
fManSyncValueInnerLoop : FLOAT; (* input value for the manual mode or the sync request *)
bSyncInnerLoop : BOOL; (* rising edge set the output to the fManSyncValue *)
eModeInnerLoop : E_CTRL_MODE; (* sets the mode of the function block *)
bHoldInnerLoop : BOOL; (* hold the internal integrator *)
fManSyncValueOuterLoop : FLOAT; (* input value for the manual mode or the sync request *)
bSyncOuterLoop : BOOL; (* rising edge set the output to the fManSyncValue *)
eModeOuterLoop : E_CTRL_MODE; (* sets the mode of the function block *)
bHoldOuterLoop : BOOL; (* hold the internal integrator *)
END_VAR
fSetpointValue : Setpoint of the controlled variable.
fActualValueOuterLoop : The actual value of the controlled variable that is fed back to the PI controller of the outer control loop.
fActualValueInnerLoop : The actual value of the controlled variable that is fed back to the PID controller of the inner control loop.
fPreControl : Pre-control that is connected behind the PI controller.
fManSyncValueInnerLoop : Input, to whose value it is possible to set the internal state of the PID element (inner control loop).
bSyncInnerLoop : A rising edge at this input sets the PID element (inner control loop) to the value fManSyncValueInnerLoop.
eModeInnerLoop : Input that specifies the operation mode of the PID element (inner control loop).
bHoldInnerLoop : A TRUE at this input holds the internal state of the PID element (inner control loop) constant at the current value.
fManSyncValueOuterLoop : Input, to whose value it is possible to set the internal state of the PI element (outer control loop).
bSyncOuterLoop : A rising edge at this input sets the PI element (the outer control loop) to the value fManSyncValueOuterLoop.
eModeOuterLoop : Input that specifies the operation mode of the PI element (the outer control loop).
bHoldOuterLoop : A TRUE at this input holds the internal state of the PI element (the outer control loop) constant at the current value.
VAR_OUTPUT
VAR_OUTPUT
fOut : FLOAT; (* manipulated value to the process *)
eStateInnerLoop : E_CTRL_STATE;
bARWactiveInnerLoop : BOOL;
eErrorIdInnerLoop : E_CTRL_ERRORCODES; (* error code *)
bErrorInnerLoop : BOOL; (* TRUE if an error situation exists *)
eStateOuterLoop : E_CTRL_STATE;
bARWactiveOuterLoop : BOOL;
eErrorIdOuterLoop : E_CTRL_ERRORCODES; (* error code *)
bErrorOuterLoop : BOOL; (* TRUE if an error situation exists *)
END_VAR
fOut : Output of the PI-PID element.
eStateInnerLoop : State of the internal PID element (inner control loop).
bARWactiveInnerLoop : A TRUE at this output indicates that the PID element (inner control loop) is being restricted.
eErrorIdInnerLoop : Returns the error number of the PID element (inner control loop) when the bError output is set.
bErrorInnerLoop : Becomes TRUE as soon as an error occurs in the PID element (inner control loop).
eStateOuterLoop : State of the internal PI element (outer control loop).
bARWactiveOuterLoop : A TRUE at this output indicates that the PID element (outer control loop) is being restricted.
eErrorIdOuterLoop : Returns the error number of the PI element (outer control loop) when the bError output is set.
bErrorOuterLoop : Is set to TRUE as soon as an error occurs in the PI element (outer control loop).
VAR_IN_OUT
VAR_IN_OUT
stParams : ST_CTRL_PI_PID_PARAMS;
END_VAR
stParams : Parameter structure of the PI-PID element. This consists of the following elements:
TYPE
ST_CTRL_PI_PID_PARAMS :
STRUCT
tCtrlCycleTime : TIME := T#0ms; (* controller cycle
time [TIME] *)
tTaskCycleTime : TIME := T#0ms; (* task cycle time
[TIME] *)
fKp_OuterLoop : FLOAT := 0; (* proportional gain
*)
tTn_OuterLoop : TIME := T#0s; (* Tn *)
fMaxLimit_OuterLoop : FLOAT := 1E38;
fMinLimit_OuterLoop : FLOAT := -1E38;
fKp_InnerLoop : FLOAT := 0; (* proportional gain
*)
tTn_InnerLoop : TIME := T#0ms; (* Tn *)
tTv_InnerLoop : TIME := T#0ms; (* Tv *)
tTd_InnerLoop : TIME := T#0ms; (* Td *)
fMaxLimit_InnerLoop : FLOAT := 1E38;
fMinLimit_InnerLoop : FLOAT := -1E38;
END_STRUCT
END_TYPE
tCtrlCycleTime : Cycle time with which the control loop is processed. This must be greater than or equal to the TaskCycleTime. The function block uses this input value to calculate internally whether the state and the output values have to be updated in the current cycle.
tTaskCycleTime : Cycle time with which the function block is called. If the function block is called in every cycle this corresponds to the task cycle time of the calling task.
fKp_OuterLoop : Controller amplification / controller coefficient of the PI element (outer control loop).
tTn_OuterLoop : Integral action time of the PI element (outer control loop). The I-component is deactivated if this is parameterized as T#0s.
fMaxLimit_OuterLoop : Upper limit at which integration of the PID element is halted and to which the output is limited (ARW measure). Reaching this limit is indicated by a TRUE at the bARWactiveOuterLoop output.
fMinLimit_OuterLoop : Lower limit at which integration of the PID element is halted and to which the output is limited (ARW measure). Reaching this limit is indicated by a TRUE at the bARWactiveOuterLoop output.
fKp_InnerLoop : Controller amplification / controller coefficient of the PID element (inner control loop).
tTn_InnerLoop : Integral action time of the PID element (inner control loop). The I-component is deactivated if this is parameterized as T#0s.
tTv_InnerLoop : Derivative action time of the PID element (inner control loop). The D-component is deactivated if this is parameterized as T#0s.
tTd_InnerLoop : Damping time of the PID element (inner control loop).
fMaxLimit_InnerLoop: Upper limit at which integration of the PID element is halted and to which the output is limited (ARW measure). Reaching this limit is indicated by a TRUE at the bARWactiveInnerLoop output.
fMinLimit_InnerLoop : Lower limit at which integration of the PID element is halted and to which the output is limited (ARW measure). Reaching this limit is indicated by a TRUE at the bARWactiveInnerLoop output.