FB_CTRL_TRANSFERFUNCTION_2
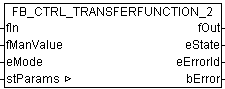
This function block calculates a discrete transfer function with the second standard form illustrated below. The transfer function here can be of any order, n.
The coefficients for the following transfer functions are stored in the parameter arrays:
Description of the transfer behavior:
The internal calculation is performed in each sampling step according to the second standard form, for which the following block diagram applies:
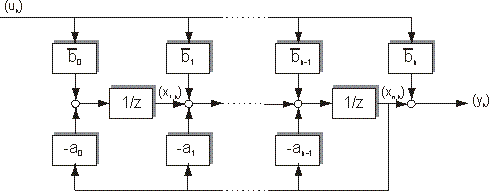
The transfer function can be brought into the form shown in the block diagram by means of some transformations:

The coefficients of the numerator polynomial are calculated according to the following rule:
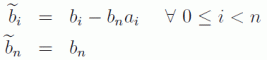
The programmer must create the following arrays in the PLC if this function block is to be used:
ar_fNumeratorArray :
ARRAY[0..nOrderOfTheTransferfunction] OF FLOAT;
ar_DenominatorArray : ARRAY[0..nOrderOfTheTransferfunction]
OF FLOAT;
ar_stTransferfunction2Data : ARRAY[0..nOrderOfTheTransferfunction]
OF ST_CTRL_TRANSFERFUNCTION_2_DATA;
The coefficients b0 to bn are stored in the array ar_fNumeratorArray. This must be organized as follows:
ar_fNumeratorArray[
0 ] := b0;
ar_fNumeratorArray[ 1 ] := b1;
...
ar_fNumeratorArray[ n-1 ] := bn-1;
ar_fNumeratorArray[ n ] := bn;
The coefficients a0 to an are stored in the array ar_DenominatorArray. This must be organized as follows:
ar_DenominatorArray
[ 0 ] := a0;
ar_DenominatorArray [ 1 ] := a1;
...
ar_DenominatorArray [ n-1 ] := an-1;
ar_DenomiantorArray [ n ] := an;
The internal data required by the function block is stored in the array ar_stTransferfunction2Data. This data must never be modified from within the PLC program. This procedure is also illustrated in the sample program listed below.
VAR_INPUT
VAR_INPUT
fIn : FLOAT;
fManValue : FLOAT;
eMode : E_CTRL_MODE;
END_VAR
fIn : Input to the transfer function.
fManValue : Input whose value is present at the output in manual mode.
eMode : Input that specifies the block's operating mode.
VAR_OUTPUT
VAR_OUTPUT
fOut : FLOAT;
eState : E_CTRL_STATE;
bError : BOOL;
eErrorId : E_CTRL_ERRORCODES;
END_VAR
fOut : Output from the transfer function.
eState : State of the function block.
eErrorId : Supplies the error number when the bError output is set.
bError : Becomes TRUE, as soon as an error occurs.
VAR_IN_OUT
VAR_IN_OUT
stParams : ST_CTRL_TRANSFERFUNCTION_2_PARAMS;
END_VAR
stParams : Parameter structure of the function block. This consists of the following elements:
TYPE
ST_CTRL_TRANSFERFUNCTION_2_PARAMS:
STRUCT
tTaskCycleTime : TIME; (* task
cycle time in seconds *)
tCtrlCycleTime : TIME := T#0ms; (*
controller cycle time *)
nOrderOfTheTransferfunction : USINT;
pNumeratorArray_ADR : POINTER TO FLOAT :=
0;
nNumeratorArray_SIZEOF : UINT;
pDenominatorArray_ADR : POINTER TO FLOAT :=
0;
nDenomiantorArray_SIZEOF : UINT;
pTransferfunction2Data_ADR : POINTER TO
ST_CTRL_TRANSFERFUNCTION_2_DATA;
nTransferfunction2Data_SIZEOF : UINT;
END_TYPE
tTaskCycleTime : Cycle time with which the function block is called. If the function block is called in every cycle this corresponds to the task cycle time of the calling task.
tCtrlCycleTime : Cycle time with which the control loop is processed. This must be greater than or equal to the TaskCycleTime. The function block uses this input value to calculate internally whether the state and the output values have to be updated in the current cycle.
nOrderOfTheTransferfunction : Order of the transfer function [0... ]
pNumeratorArray_ADR : Address of the array with the numerator coefficients.
nNumeratorArray_SIZEOF : Size of the array with the numerator coefficients in bytes.
pDenominatorArray_ADR : Address of the array with the denominator coefficients.
nDenominatorArray_SIZEOF : Size of the array with the denominator coefficients in bytes.
pTransferfunction2Data_ADR : Address of the data array.
nTransferfunction2Data_SIZEOF : Size of the data array in bytes.
TYPE
ST_CTRL_TRANSFERFUNCTION_2_DATA:
STRUCT
Interne Struktur. Auf diese darf nicht schreibend
zugegriffen werden.
END_STRUCT
END_TYPE
Sample:
PROGRAM PRG_TRANSFERFUNCTION_2_TEST
VAR CONSTANT
nOrderOfTheTransferfunction : USINT := 2;
END_VAR
VAR
ar_fNumeratorArray :
ARRAY[0..nOrderOfTheTransferfunction] OF FLOAT;
ar_DenominatorArray :
ARRAY[0..nOrderOfTheTransferfunction] OF FLOAT;
ar_stTransferfunction2Data :
ARRAY[0..nOrderOfTheTransferfunction] OF
ST_CTRL_TRANSFERFUNCTION_2_DATA;
eMode : E_CTRL_MODE;
stParams : ST_CTRL_TRANSFERFUNCTION_2_PARAMS;
eErrorId : E_CTRL_ERRORCODES;
bError : BOOL;
fbTansferfunction : FB_CTRL_TRANSFERFUNCTION_2;
bInit : BOOL := TRUE;
fIn : FLOAT := 0;
fOut : FLOAT;
b_0, b_1, b_2 : FLOAT;
a_0,a_1,a_2 : FLOAT;
END_VAR
IF bInit
THEN
(* set values in the local arrays *)
ar_fNumeratorArray[0] := 1.24906304658218E-007;
ar_fNumeratorArray[1] := 2.49812609316437E-007;
ar_fNumeratorArray[2] := 1.24906304658218E-007;
ar_DenominatorArray[0] := 0.998501124344101;
ar_DenominatorArray[1] := -1.99850062471888;
ar_DenominatorArray[2] := 1.0;
(* set values in the parameter struct *)
stParams.tTaskCycleTime := T#2ms;
stParams.tCtrlCycleTime := T#2ms;
stParams.nOrderOfTheTransferfunction :=
nOrderOfTheTransferfunction;
(* set the mode *)
eMode := eCTRL_MODE_ACTIVE;
bInit := FALSE;
END_IF
(* set the addresses *)
stParams.pNumeratorArray_ADR := ADR( ar_fNumeratorArray);
stParams.nNumeratorArray_SIZEOF := SIZEOF(
ar_fNumeratorArray);
stParams.pDenominatorArray_ADR := ADR( ar_DenominatorArray );
stParams.nDenominatorArray_SIZEOF := SIZEOF( ar_DenominatorArray
);
stParams.pTransferfunction2Data_ADR := ADR(
ar_stTransferfunction2Data );
stParams.nTransferfunction2Data_SIZEOF := SIZEOF(
ar_stTransferfunction2Data );
fbTansferfunction ( fIn := fIn,
eMode := eMode,
stParams := stParams,
fOut => fOut,
eErrorId => eErrorId,
bError => bError
);