FB_CTRL_LOOP_SCHEDULER
This function block allows the system loading to be distributed over a number of control loops that are a) parameterised using the same tCtrlCycleTime and b) for which the condition tCtrlCycleTime > tTaskCycleTime is true. The output vector calculated by this block is used to start the individual control loops at different times, so that the system loading is distributed.
Behavior of the output vector:
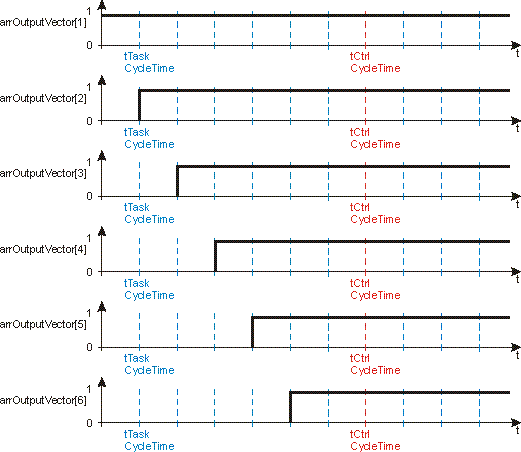
6 control loops are managed in this diagram. In this case, tCtrlCycleTime = 7 · tTaskCycleTime.
The programmer must create the following array in the PLC if this function block is to be used:
arrOutputVector :
ARRAY[1..nNumberOfControlLoops] OF BOOL;
The function block sets the bits in this vector to TRUE or FALSE. The control loops that are managed with the loop scheduler are to be switched into the eCTRL_MODE_ACTIVE state when the corresponding bit in the output vector is TRUE. See sample code below.
VAR_INPUT
VAR_INPUT
nManValue : DWORD;
eMode : E_CTRL_MODE;
END_VAR
nManValue : This input allows the first 32 bits in the output vector to be set in eCTRL_MODE_MANUAL. A 1 sets the first bit, a 2 the second bit, a 3 the first and second bits, ...
eMode : Input that specifies the operation mode of the function block.
VAR_OUTPUT
VAR_OUTPUT
eState : E_CTRL_STATE;
eErrorId : E_CTRL_ERRORCODES;
bError : BOOL;
END_VAR
eState : State of the function block.
nErrorID : Returns the ADS error number when the bError output is set.
bError: Becomes TRUE, as soon as an error occurs.
VAR_IN_OUT
VAR_IN_OUT
stParams : ST_CTRL_LOOP_SCHEDULER_PARAMS;
END_VAR
stParams : Parameter structure for the loop scheduler. This consists of the following elements:
TYPE
ST_CTRL_LOOP_SCHEDULER_PARAMS:
STRUCT
tCtrlCycleTime : TIME := T#0ms; (*
controller cycle time [TIME] *)
tTaskCycleTime : TIME := T#0ms; (* task
cycle time [TIME] *)
nNumberOfControlLoops : UINT;
pOutputVector_ADR : POINTER TO BOOL := 0;
nOutputVector_SIZEOF : UINT := 0;
END_STRUCT
END_TYPE
tCtrlCycleTime : The cycle time with which the control loops managed by the loop scheduler are processed. This must be greater than or equal to the TaskCycleTime.
tTaskCycleTime : The cycle time with which the loop scheduler and the function blocks associated with the control loops are called. If the block is called in every cycle this corresponds to the task cycle time of the calling task.
nNumberOfControlLoops : The number of control loops being managed.
pOutputVector_ADR :.Address of the output vector.
nOutputVector_SIZEOF :Size of the output vector in bytes.
Sample:
PROGRAM PRG_LoopScheduler
VAR
arrOutputVector : ARRAY[1..5] OF BOOL;
eMode : E_CTRL_MODE;
stParams : ST_CTRL_LOOP_SCHEDULER_PARAMS;
eErrorId : E_CTRL_ERRORCODES;
bError : BOOL;
fbCTRL_LoopScheduler : FB_CTRL_LOOP_SCHEDULER;
bInit : BOOL := TRUE;
(* modes of the control loops *)
eMode_CtrlLoop_1 : E_CTRL_MODE;
eMode_CtrlLoop_2 : E_CTRL_MODE;
eMode_CtrlLoop_3 : E_CTRL_MODE;
eMode_CtrlLoop_4 : E_CTRL_MODE;
eMode_CtrlLoop_5 : E_CTRL_MODE;
END_VAR
IF bInit
THEN
stParams.tCtrlCycleTime := T#10ms;
stParams.tTaskCycleTime := T#2ms;
stParams.nNumberOfControlLoops := 5;
bInit := FALSE;
END_IF
(* set addresses *)
stParams.nOutputVector_SIZEOF := SIZEOF(arrOutputVector);
stParams.pOutputVector_ADR := ADR(arrOutputVector);
(* call scheduler *)
fbCTRL_LoopScheduler( eMode := eMode,
stParams := stParams,
eErrorId => eErrorId,
bError => bError);
IF arrOutputVector[ 1 ]
THEN
(* activate control loop 1 *)
eMode_CtrlLoop_1 := eCTRL_MODE_ACTIVE;
END_IF
IF arrOutputVector[ 2 ]
THEN
(* activate control loop 2 *)
eMode_CtrlLoop_2 := eCTRL_MODE_ACTIVE;
END_IF
IF arrOutputVector[ 3 ]
THEN
(* activate control loop 3 *)
eMode_CtrlLoop_3 := eCTRL_MODE_ACTIVE;
END_IF
IF arrOutputVector[ 4 ]
THEN
(* activate control loop 4 *)
eMode_CtrlLoop_4 := eCTRL_MODE_ACTIVE;
END_IF
IF arrOutputVector[ 5 ]
THEN
(* activate control loop 5 *)
eMode_CtrlLoop_5 := eCTRL_MODE_ACTIVE;
END_IF