FB_EnumStringNumbers
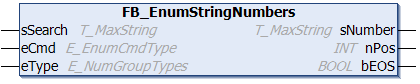
This function block can be used to search a string in a REPEAT or WHILE loop for numbers. The string may contain several numbers. Any numbers that are found are output as sub-strings at the function block output. The function searches from the current position for the first character that can be interpreted as a numeral. The search is aborted if a character is found that cannot be interpreted as a number. The eCmd parameter determines whether the search is for the first number or the next number. The eType parameter determines the format of the numbers in the search string.
Inputs
VAR_INPUT
sSearch : T_MaxString;
eCmd : E_EnumCmdType := eEnumCmd_First;
eType : E_NumGroupTypes := eNumGroup_Float;
END_VAR
Name | Type | Description |
---|---|---|
sSearch | T_MaxString | Search string to be searched for numbers |
eCmd | Control command for the enumeration block | |
eType | Number format of the searched number. This parameter determines which characters are to be ignored and which are to be interpreted as numerals: |
Value | Meaning |
---|---|
eNumGroup_Float | Numbers '0' to '9', '+', '-' (signs) and 'e' or 'E' (exponent character) are interpreted as valid numerals. |
eNumGroup_Unsigned | Numbers '0' to '9' are interpreted as valid numerals. '+', '-', 'e', 'E' characters are ignored as numerals. |
eNumGroup_Signed | Numbers '0' to '9', '+', '-' (signs) are interpreted as valid numerals. 'e', 'E' characters are ignored. |
If the string contains numbers in the exponential notation, eType = eNumGroup_Float must be set (default).
Outputs
VAR_OUTPUT
sNumber : T_MaxString;
nPos : INT;
bEOS : BOOL;
END_VAR
Name | Type | Description |
---|---|---|
sNumber | T_MaxString | The last found number as string |
nPos | INT | This variable always returns the position after the last found and correctly formatted number character. I.e. at this point the function block begins to search for new number characters at the next call. nPos is zero if the final zero of the sSearch string has been reached. The first character in the string has position number = 1 (non-zero based position). |
bEOS | BOOL | This variable is FALSE if a new number was found and the end of the string has not yet been reached. In this case, sNumber returns a valid number as a string. This variable is TRUE if no other number was found. In this case any further search must be aborted (sNumber returns no valid value). |
Example:
In the following example the sNumber variable is searched for valid numbers. Any sub-strings that are found are stored in the array variable arrNums.
TYPE ST_ScanRes :
STRUCT
sNumber : T_MaxString;
nPos : INT;
sRemain : T_MaxString;
END_STRUCT
END_TYPE
PROGRAM MAIN
VAR
sSearch : T_MaxString := 'Some numbers in string: +-12e-34, -56, +78';
fbEnum : FB_EnumStringNumbers := ( eType := eNumGroup_Float (* eNumGroup_Signed, eNumGroup_Unsigned *) );
arrNums : ARRAY[1..MAX_SCAN_NUMS] OF ST_ScanRes;
idx : INT;
length : INT;
bEnum : BOOL := TRUE;
END_VAR
VAR CONSTANT
MAX_SCAN_NUMS : INT := 10;
END_VAR
IF bEnum THEN
bEnum := FALSE;
MEMSET( ADR( arrNums ), 0, SIZEOF( arrNums ) );
idx := 0;
length := LEN( sSearch );
fbEnum( sSearch := sSearch, eCmd := eEnumCmd_First );
WHILE NOT fbEnum.bEOS DO
IF idx < MAX_SCAN_NUMS THEN
idx := idx + 1;
arrNums[idx].sNumber:= fbEnum.sNumber;
arrNums[idx].nPos := fbEnum.nPos;
IF fbEnum.nPos <> 0 THEN
arrNums[idx].sRemain:= RIGHT( sSearch, length - fbEnum.nPos + 1 );
END_IF
END_IF
fbEnum( eCmd := eEnumCmd_Next );
END_WHILE
END_IF
The found strings:
- '-12e-34'
- '-56'
- '+78'
eType parameter eNumGroup_Signed returns the following results:
- '-12'
- '-34'
- '-56'
- '+78'
eType parameter eNumGroup_Unsigned returns the following results:
- '12'
- '34'
- '56'
- '78'
Requirements
Development environment | Target platform | PLC libraries to be integrated (category group) |
---|---|---|
TwinCAT v3.1.0 | PC or CX (x86, x64, Arm®) | Tc2_Utilities (System) |