FB_CoEWrite_ByDriveRef
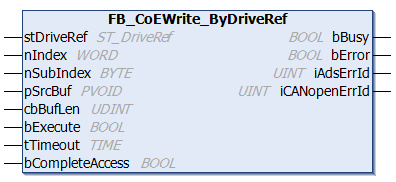
The function block FB_CoEWrite_ByDriveRef
can be used to write drive parameters based on the “CANopen over EtherCAT (CoE)” protocol. This requires the slave to have a mailbox and to support the “CANopen over EtherCAT” (CoE) protocol. The nSubIndex and nIndex parameters allow the object that is to be written to be selected. Via bCompleteAccess := TRUE
the parameter can be written with sub-elements.
Inputs
VAR_INPUT
stDriveRef : ST_DriveRef; (*Contains sNetID of EcMaster, nSlaveAddr EcDrive, nDriveNo of EcDrive, either preset or read from NC*)
nIndex : WORD; (*SoE IDN: e.g. “S_0_IDN+1” for S-0-0001 or “P_0_IDN+23” for P-0-0023*)
nSubIndex : BYTE; (*SoE element*)
pSrcBuf : PVOID; (*Contains the address of the buffer containing the data to be sent*)
cbBufLen : UDINT; (*Contains the max. number of bytes to be received*)
bExecute : BOOL; (*Function block execution is triggered by a rising edge at this input*)
tTimeout : TIME; (*States the time before the function is cancelled*)
bCompleteAccess : BOOL;
END_VAR
Name | Type | Description |
---|---|---|
stDriveRef | ST_DriveRef | Structure containing the AMS network ID of the EtherCAT master device and the address of the slave device. The reference to the drive can be linked directly to the PLC in the System Manager. To this end an instance of ST_PlcDriveRef must be used and the NEtID of the Byte array converted to a string. |
nIndex | WORD | Index of the object that is supposed to be written. |
nSubIndex | BYTE | Subindex of the object that is supposed to be written. |
pSrcBuf | PVOID | Address (pointer) to the transmit buffer |
cbBufLen | UDINT | Maximum available buffer size for the data to be sent in bytes. |
bExecute | BOOL | The function block is activated by a positive edge at this input. |
tTimeout | TIME | Maximum time allowed for the execution of the function block. |
bComplete | BOOL | If bCompleteAccess is set, the whole parameter can be read in a single access. |
Outputs
VAR_OUTPUT
bBusy : BOOL;
bError : BOOL;
iAdsErrId : UINT;
iCANopenErrId : UINT;
END_VAR
Name | Type | Description |
---|---|---|
bBusy | BOOL | This output is set when the function block is activated, and remains set until a feedback is received. |
bError | BOOL | This output is set after the bBusy output has been reset when an error occurs in the transmission of the command. |
iAdsErrId | UINT | Returns the ADS error code of the last executed command when the bError output is set. |
iCANopenErrId | UINT | Returns the CANopen error code if the bError output is set. |
Example of an implementation in ST:
PROGRAM MAIN
VAR
fbCoEWrite : FB_CoEWrite_ByDriveRef;
stDriveRef : ST_DriveRef;
nIndex : WORD := 16#1018;
nSubIndex : BYTE := 1;
bExecute : BOOL := TRUE;
tTimeout : TIME := T#5S;
bCompleteAccess : BOOL := TRUE;
vendorId : UDINT := 2;
bError : BOOL;
nAdsErrId : UDINT;
nCANopenErrId : UDINT;
END_VAR
fbCoEWrite(
stDriveRef:= stDriveRef,
nIndex:= nIndex,
nSubIndex:= nSubIndex,
pSrcBuf:= ADR(vendorId),
cbBufLen:= SIZEOF(vendorId),
bExecute:= bExecute,
tTimeout:= tTimeout,
bCompleteAccess:= bCompleteAccess,
);
IF NOT fbCoEWrite.bBusy THEN
bError:= fbCoEWrite.bError;
nAdsErrId:= fbCoEWrite.iAdsErrId;
nCANopenErrId:= fbCoEWrite.iCANopenErrId;
bExecute := FALSE;
fbCoEWrite(bExecute := bExecute);
END_IF
Requirements
Development environment | Target platform | PLC libraries to include |
---|---|---|
TwinCAT v3.1.0 | PC or CX (x86, x64, ARM) | Tc2_EtherCAT |