Project Components
A project contains all objects of a control program. A project is saved in a file with the name of the project. A project includes the following objects:
function blocks, data types, resources and libraries.
POU (Program Organization Unit)
Functions, function blocks, and programs are POUs, which can be supplemented by actions.
Each POU consists of a declaration part and a body. The body is written in one of the IEC programming languages which include IL, ST, SFC, FBD, LD or CFC. TwinCAT PLC Control supports all IEC standard POUs. If you want to use these POUs in your project, you must include the library standard.lib in your project.
POUs can call up other POUs. However, recursions are not allowed.
Function
A function is a function block that returns exactly one data element (which can also be multi-element, such as fields or structures) when executed, and whose call in textual languages can occur as an operator in expressions.
When declaring a function, it is important to note that the function must be given a type. I.e. after the function name a colon followed by a type must be entered.
A correct function declaration looks like this, for example:
FUNCTION Fct: INT
In addition, a result must be assigned to the function. The function name is used like an output variable.
Sample in IL for a function that takes three input variables and returns as result the product of the first two divided by the last one:
The call to a function can occur in ST as an operand in expressions.
Functions do not have internal states. I.e. calls of a function with the same arguments (input parameters) always return the same value (output).
![]() | If a local variable is declared as RETAIN in a function, this has no effect. The variable is not stored in the retain area. |
Samples of calling the function described above:
in IL:
LD 7
Fct 2,4
ST Ergebnis
in ST:
Ergebnis := Fct(7, 2, 4);
in FBD:
In SFC, a function call can only be made within a step or transition.
If you define a function in your project with the name CheckBounds, you can use it to check for range overflows in your project! The name of the function is defined and may have only this identifier.
The following typical program for testing the CheckBounds function goes beyond the boundaries of a defined array. The CheckBounds functions makes sure that the value TRUE is not assigned to the position A[10], but rather to the upper area boundary A[7] which is still valid. Therefore, the CheckBounds function can be used to correct extensions beyond array boundaries.
If you define functions in your project with the name CheckDivByte, CheckDivWord, CheckDivDWord and CheckDivReal, you can use them to check the value of the divisor if you use the operator DIV, for example to avoid a division by 0. The name of the function is defined and may have only this identifier.
If you define functions with the names CheckRangeSigned and CheckRangeUnsigned, then range exceeding of variables declared wit subrange types can be intercepted.
All these check function names are reserved for the described usage.
Function block
A function block is a POU which provides one or more values during the procedure. As opposed to a function, a function block provides no return value.
Example in IL of a function block with two input variables and two output variables. One output is the product of the two inputs, the other a comparison for equality:
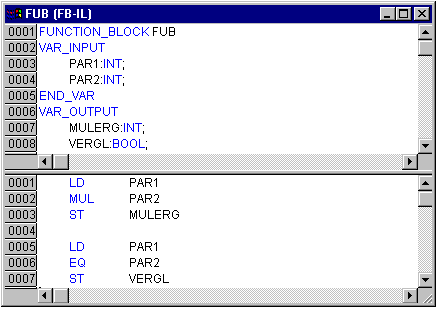
Function block Instances
Reproductions or instances (copies) of a function block can be created. Each instance possesses its own identifier (the Instance name), and a data structure which contains its inputs, outputs, and internal variables. Instances are declared locally or globally as variables, whereas the name of the function block is indicated as the type of an identifier.
Example of an instance with the name INSTANCE of the FUB function block:
INSTANCE: FUB;
Function blocks are always called through the instances described above.
Only the input and output parameters can be accessed from outside of a function block instance, not its internal variables.
Example for accessing an input variable: The function block FB has an input variable in1 of the type INT.
PROGRAM prog
VAR
inst1:fb;
END_VAR
LD 17
ST inst1.in1
CAL inst1
END_PROGRAM
The declaration parts of function blocks and programs can contain instance declarations. Instance declarations are not permitted in functions.
Access to a function block instance is limited to the POU in which it was declared unless it was declared globally. The instance name of a function block instance can be used as the input for a function or a function block.
![]() | All values are retained from one execution of the function block to the next. Therefore, calls of a function block with the same arguments do not always return the same output values! |
![]() | If the function block contains at least one retain variable, the entire instance is stored in the retain area. |
Calling a function block
The input and output variables of a function block can be accessed from another POU by setting up an instance of the function block and specifying the desired variable using the following syntax:
<Instance name>.<Variable name>
If you would like to set the input parameters (thus the value of the inputvariables) when you open the function block, you can do this in the text languages IL and ST by assigning values to the parameters after the instance name of the function block in parentheses (this assignment takes place using ":=" just as with the initialization of variables at the declaration position).
Please note, that the input/output variables (VAR_IN_OUT) of a POU will be turn over as a pionter. Therefore no constants can be assigned to them at a call, and an external read or write access is impossible.
Example for the call of a VAR_IN_OUT variable inout1 of the POU fubo in ST:
VAR
inst:fubo;
var1:int;
END_VAR
var1:=2;
inst(inout1:=var1);
Not allowed: inst(inout1:=2); or. inst.inout1:=2;
Examples for calling function block FUB described above. The multiplication result is saved in the variable ERG, and the result of the comparison is saved in QUAD. An instance of FUB with the name INSTANCE is declared:
In IL the function block is called as follows:
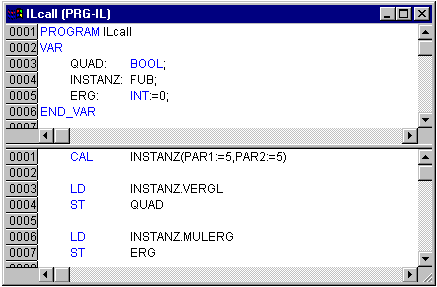
In the example below the call is shown in ST. The declaration part is the same as with IL:
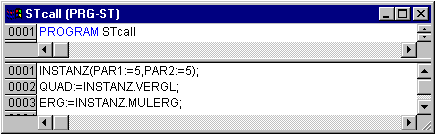
In FBD it would look as follows (declaration part the same as with IL):
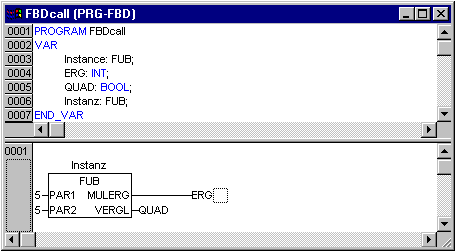
In SFC function block calls can only take place in steps.
Program
A program is a POU which returns several values during operation. Programs are recognized globally throughout the project. All values are retained from the last time the program was run until the next.
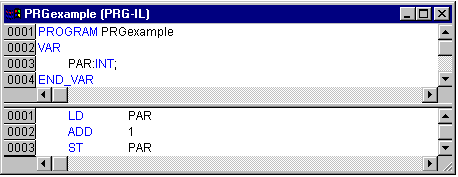
Programs can be called. A program call in a function is not allowed. There are also no instances of programs. If a POU calls a program, and if thereby values of the program are changed, then these changes are retained the next time the program is called, even if the program has been called from within another POU. This is different from calling a function block. There only the values in the given instance of a function block are changed. These changes therefore play a role only when the same instance is called. A program declaration begins with the keyword PROGRAM and ends with END_PROGRAM.
Examples of calls of the program described above:
In IL:
CAL PRG Example
LD PRGexample.PAR
ST ERG
in ST:
PRGExample;
Erg := PRGexample.PAR;
In FBD:
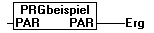
If the variable PAR from the program PRGexample is initialized by a main program with 0, and then one after the other programs are called with above named program calls, then the ERG result in the programs will have the values 1, 2, and 3. If one exchanges the sequence of the calls, then the values of the given result parameters also change in a corresponding fashion.
Action
As addition to function blocks and programs you can define actions. An action is an further implementation. It can be written in a different language than the 'normal' implementation. Each action gets a name.
An action works with the data of the accompanying function block or program. It uses the same input/output variables and local variables like the 'normal' implementation..
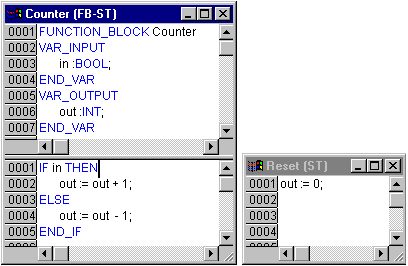
When function block Counter is called, the output variable gets increased or decreased depending on the input variable 'in'. When action Reset, affiliated to the function block is called, the output variable is set to 0. In both cases the same variable out is written.
An action is called with <program name>.<action name> respectively <instance name>.<action name>. If the action should be called within the 'mother' module, in text editors only the action name is used, in graphical editors the function block call without instance name.
Example
Declaration for all Examples:
PROGRAM PLC_PRG
VAR
Inst : Counter;
END_VAR
In AWL:
CAL Inst.Reset(In := FALSE)
LD Inst.out
ST ERG
In ST:
Inst.Reset(In := FALSE);
Erg := Inst.out;
In FBD:
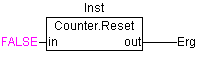
See Chapter SFC for more information about SFC Actions.
In IEC 61131-3 there are only actions for SFC described.
Ressourcen
You need the ressources for configuring and organizing your project and for tracing variable values:
- Global Variables which can be used throughout the project
- PLC Configuration for configuring your hardware
- Task Configuration for guiding your program through tasks
- Sampling Trace for graphic display of variable values
- Watch and Recepe Manager for displaying variable values and setting default variable values
See the chapter called "The Ressources".
Libraries
You can include in your project a series of libraries whose POUs, data types, and global variables you can use just like user-defined variables. The library "standard.lib" is a standard part of the program and is always at your disposal.
See the chapter called "Library Manager".
Data types
Along with the standard data types the user can define his own data types. Structures, enumeration types and references can be created.
See 'Standard-' and 'User Defined Datatypes' in the appendix.