Custom TreeItem Parameters
The ITcSmTreeItem interface is supported by every TwinCAT tree item and has a very generic character. To support the specification of all the devices, boxes and terminals, together with many other different types of tree items, all custom parameters of a tree item are accessible via the XML representation of the tree item. This XML-String can be accessed by the method ITcSmTreeItem::ProduceXml and its counterpart ITcSmTreeItem::ConsumeXml. This function pair has the same functionality as the "Export XML Description ..." and "Import XML Description ..." commands from the main menu of the TwinCAT IDE (see snapshot below).
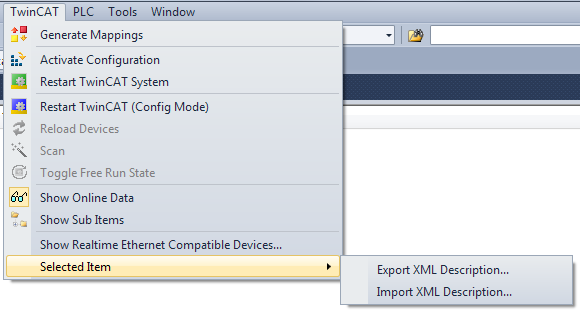
With this Import/Export functionset, good parts of any script or automation code can be tested and tailored conveniently, before it is developed within the coding language - simply by the process of exporting tree item data, changing its content and re-importing it.
Best practice is to export the XML content first, change its content, importing it within the IDE and then, if everything goes successfully, package it into the programming/script code for handling it via the methods ProduceXml and ConsumeXml.
Using this workflow ensures that all changed properties and methods are valid (the Xml stream is self-documenting) and in which way they can be used (for example as Read-Only-Property, as Read-Write-Property or as a method trigger).
Reading Properties
In this example, the Network Adapter setting of an TwinCAT configuration should be detected (here an CX9001): Here the Adapter Settings are available within the DeviceDef specific part of the XML Data.
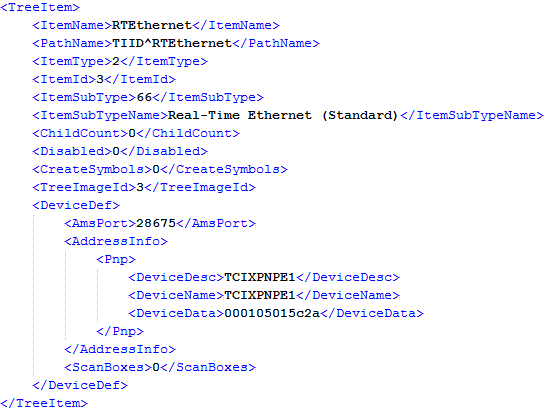
The Adapter's MAC-Address can be found at the XmlPath "TreeItem/DeviceDef/AddressInfo/Pnp/DeviceData". With this information the script code that reads this information can be written:
Code Snippet (IronPython) for TwinCAT 3:
import System
import clr
import System.IO;
clr.AddReference('System.Xml')
from System.Xml import XmlDocument, XmlTextReader
from System.IO import File,FileInfo, Directory, DirectoryInfo
#create Visual Studio
t = System.Type.GetTypeFromProgID("VisualStudio.DTE.10.0");
dte = System.Activator.CreateInstance(t)
solution = dte.Solution
if (Directory.Exists(r"C:\SolutionFolder")):
Directory.Delete(r"C:\SolutionFolder",True)
Directory.CreateDirectory(r"C:\SolutionFolder")
Directory.CreateDirectory(r"C:\SolutionFolder\MySolution1");
solution.Create(r"C:\SolutionFolder","MySolution1")
solution.SaveAs(r"C:\SolutionFolder\MySolution1\MySolution1.sln")
template = r"C:\TwinCAT3\Components\Base\PrjTemplate\TwinCAT Project.tsp"
project = solution.AddFromTemplate(template,"C:\SolutionFolder\MySolution1","MyProject")
#Get the Specific System Manager Interface
sysMan = project.Object
sysMan = project.Object
ioDevices = sysMan.LookupTreeItem("TIID")
treeItem = ioDevices.CreateChild("RTEthernet",66,"",None) #Adding the RT-Ethernet Device
xml = treeItem.ProduceXml()
xmlDoc = XmlDocument()
xmlDoc.LoadXml(xml)
test = xmlDoc.SelectSingleNode("TreeItem/DeviceDef/AddressInfo/Pnp/DeviceData")
deviceDataString = test.InnerText;
print deviceDataString # Print the MacID of the Device (contains 000105015c2a here)
Writing Properties
In this example, the Adapter settings should also be written to the configuration. Shortening the original XML to the following form and changing the Adapter parameters, will activate the new parameter set within the configuration (as long the parameters are correct). To test the settings before writing the script code, the "Import Xml Descriptions" Menu function is very helpful.
After the parameter change has been tested from within TwinCAT XAE, it is easy to write the script code that creates the valid parameterset and feeds the ITcTreeItem via the method ITcTreeItem::ConsumeXml(xml), for example:
xml = '<TreeItem> \
<DeviceDef>\
<AddressInfo>\
<Pnp>\
<DeviceDesc>TCIXPNPE2</DeviceDesc>\
<DeviceName>TCIXPNPE2</DeviceName>\
<DeviceData>00010501958f</DeviceData>\
</Pnp>\
</AddressInfo>\
</DeviceDef>\
</TreeItem>'
device = sysMan.LookupTreeItem("TIID^RTEthernet")
device.ConsumeXml(xml)
Calling Methods
In some cases, specific methods should be triggered, for example the ScanBoxes functionality from TwinCAT XAE, which scans the device for all connected boxes. Looking at the XML-example from the above chapter Reading-Properties, the XML-Content contains a flag <ScanBoxes>0</ScanBoxes>. This sort of XmlElement is called a "Method Trigger" and should indicate that the "ScanBoxes" functionality is currently not triggered. Consuming the following XML-Code at the device item will start a "ScanBoxes" and insert all found Boxes / Terminals into the configuration.
Xml Data:
Code Snippet (IronPython):
xml = '<TreeItem> \
<DeviceDef>\
<ScanBoxes>1</ScanBoxes>\
</DeviceDef>\
</TreeItem>'
device = sysMan.LookupTreeItem("TIID^RTEthernet")
device.ConsumeXml(xml)