Reading and writing string variables
Requirements
Visual Studio 2010
Silverlight 4 Tools
TwinCAT ADS WCF
Description
Read a string from a plc variable and display it in the visualization or write a string from the visualization to a plc variable.
The TwinCAT.Ads.SL DLL (Silverlight) is used to read the data from the recieved byte array in an easy to handle way.
While using the WCF service within a Silverlight application, all methods of the used context interface are appended with the postfix Async.
And there is a extra callback method for each method with the postfix Completed too.
Example: Read1 -> Read1Async, Read1Completed
This is important because silverlight allows asynchronous communication only. A read action by the use of the Read1 method is requested by the use of the Read1Async method and the response will raise the Read1Completed callback.
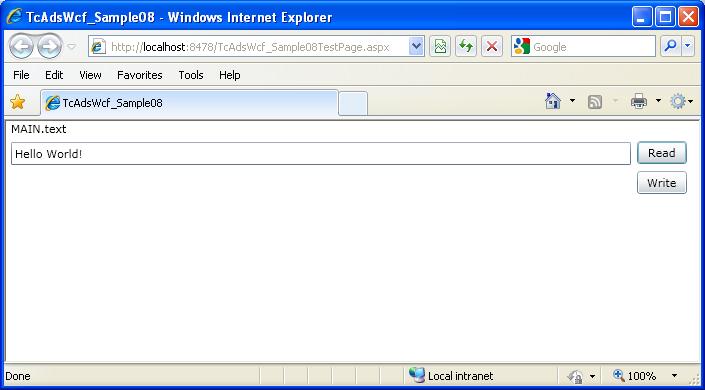
Silverlight 4 application
using System;
using System.ServiceModel;
using System.Windows;
using System.Windows.Controls;
using TcAdsWcf_Sample08.TwinCAT.Ads.Wcf;
using TwinCAT.Ads.SL;
namespace TcAdsWcf_Sample08
{
public partial class MainPage : UserControl
{
// If the netid string is empty, the TwinCAT ADS WCF service will use the TwinCAT Runtime on its host machine.
private const string NETID = "";
private const int PORT = 801;
private TcAdsServiceSimplexClient wcfClient;
public MainPage ( )
{
InitializeComponent ( );
wcfClient = new TcAdsServiceSimplexClient (
new BasicHttpBinding ( ),
new EndpointAddress ( "http://localhost:8003/TwinCAT/Ads/Wcf/TcAdsService/UnsecBasicHttp" ));
wcfClient.Read1Completed += new EventHandler<Read1CompletedEventArgs1>(wcfClient_Read1Completed);
}
private void wcfClient_Read1Completed(object sender, Read1CompletedEventArgs1 e)
{
// Create AdsStream and AdsBinaryReader for buffer handling.
AdsStream stream = new AdsStream(e.Result);
AdsBinaryReader binReader = new AdsBinaryReader(stream);
stream.Position = 0;
tbText.Text = binReader.ReadPlcString(30);
}
private void btnRead_Click ( object sender, RoutedEventArgs e )
{
// Read text asynchronous.
wcfClient.Read1Async ( NETID, PORT, "MAIN.text", 30, null );
}
private void btnWrite_Click ( object sender, RoutedEventArgs e )
{
// Create a byte array buffer.
// Length of text +1 because of zero termination.
byte [ ] buffer = new byte [ tbText.Text.Length + 1 ];
// Initialize an AdsStream and an AdsBinaryWriter to write the text to the buffer.
AdsStream stream = new AdsStream ( buffer );
AdsBinaryWriter binWriter = new AdsBinaryWriter ( stream );
binWriter.WritePlcString ( tbText.Text, tbText.Text.Length + 1 );
// Send the buffer.
wcfClient.Write1Async ( NETID, PORT, "MAIN.text", buffer );
}
}
}