Event driven reading
Requirements
Visual Studio 2010
Silverlight 4 Tools
TwinCAT ADS WCF 1.1.0.1
Description
Reading symbol data from the PLC by ADS notification callback on symbol change.
The TwinCAT.Ads.SL DLL (Silverlight) is used to read the data from the recieved byte array in an easy to handle way.
While using the WCF service within a Silverlight application, all methods of the used context interface are appended with the postfix Async.
And there is a extra callback method for each method with the postfix Completed too.
Example: Read1 -> Read1Async, Read1Completed
This is important because silverlight allows asynchronous communication only. A read action by the use of the Read1 method is requested by the use of the Read1Async method and the response will raise the Read1Completed callback.
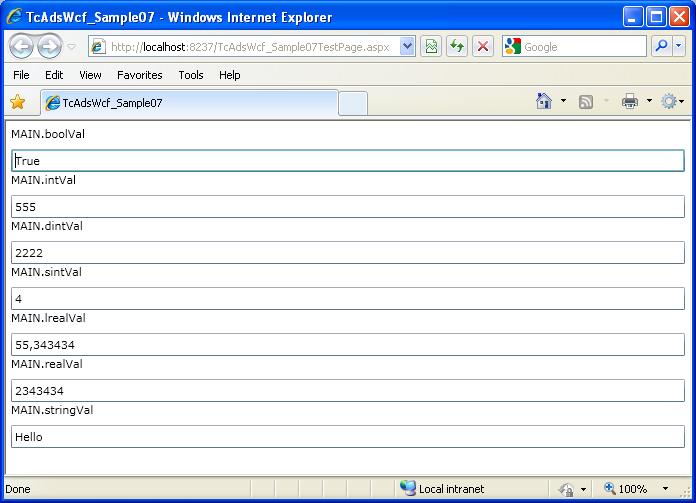
Silverlight 4 application
using System;
using System.ServiceModel;
using System.ServiceModel.Channels;
using System.Windows.Controls;
using TcAdsWcf_Sample07.TwinCAT.Ads.Wcf;
using TwinCAT.Ads.SL;
namespace TcAdsWcf_Sample07
{
public partial class MainPage : UserControl
{
// If the netid string is empty, the TwinCAT ADS WCF service will use the TwinCAT Runtime on its host machine.
private const string NETID = "";
private const int PORT = 801;
private TcAdsServiceDuplexClient wcfClient;
private int[] handles = new int [ 7 ];
public MainPage ( )
{
InitializeComponent ( );
// Initialize WCF client.
wcfClient = new TcAdsServiceDuplexClient(
new PollingDuplexHttpBinding(
PollingDuplexHttpSecurityMode.None,
PollingDuplexMode.MultipleMessagesPerPoll ),
new EndpointAddress ( "http://localhost:8003/TwinCAT/Ads/Wcf/TcAdsService/UnsecPollingDuplex4" ) );
// Register asynchronous EventHandler.
wcfClient.AddDeviceNotification1Completed += new EventHandler<AddDeviceNotification1CompletedEventArgs> ( wcfClient_AddDeviceNotification1Completed );
// Register CallbackChannel EventHandler.
wcfClient.AdsNotificationReceived += new EventHandler<AdsNotificationReceivedEventArgs> ( wcfClient_AdsNotificationReceived );
// Subscribe to the AdsNotificationEvent.
wcfClient.SubscribeAdsNotificationEventAsync ( NETID, PORT );
// Add device notifications.
wcfClient.AddDeviceNotification1Async (
NETID, PORT, "MAIN.boolVal", 1, AdsTransMode.OnChange, 100, 0, null, 0 );
wcfClient.AddDeviceNotification1Async (
NETID, PORT, "MAIN.intVal", 2, AdsTransMode.OnChange, 100, 0, null, 1 );
wcfClient.AddDeviceNotification1Async (
NETID, PORT, "MAIN.dintVal", 4, AdsTransMode.OnChange, 100, 0, null, 2 );
wcfClient.AddDeviceNotification1Async (
NETID, PORT, "MAIN.sintVal", 1, AdsTransMode.OnChange, 100, 0, null, 3 );
wcfClient.AddDeviceNotification1Async (
NETID, PORT, "MAIN.lrealVal", 8, AdsTransMode.OnChange, 100, 0, null, 4 );
wcfClient.AddDeviceNotification1Async (
NETID, PORT, "MAIN.realVal", 4, AdsTransMode.OnChange, 100, 0, null, 5 );
wcfClient.AddDeviceNotification1Async (
NETID, PORT, "MAIN.stringVal", 50, AdsTransMode.OnChange, 100, 0, null, 6 );
}
private void wcfClient_AddDeviceNotification1Completed ( object sender, AddDeviceNotification1CompletedEventArgs e )
{
int index = ( int ) e.UserState;
handles [ index ] = e.Result;
}
private void wcfClient_AdsNotificationReceived ( object sender, AdsNotificationReceivedEventArgs e )
{
// Create AdsStream and AdsBinaryReader for buffer handling.
AdsStream adsStream = new AdsStream ( e.buffer );
AdsBinaryReader binReader = new AdsBinaryReader ( adsStream );
adsStream.Position = e.offset;
// Identify notification by handle and update GUI.
if ( e.notificationHandle == handles [ 0 ] )
{
textBoxBool.Text = binReader.ReadBoolean ( ).ToString ( );
}
else if ( e.notificationHandle == handles [ 1 ] )
{
textBoxInt.Text = binReader.ReadInt16 ( ).ToString ( );
}
else if ( e.notificationHandle == handles [ 2 ] )
{
textBoxDint.Text = binReader.ReadInt32 ( ).ToString ( );
}
else if ( e.notificationHandle == handles [ 3 ] )
{
textBoxSint.Text = binReader.ReadByte ( ).ToString ( );
}
else if ( e.notificationHandle == handles [ 4 ] )
{
textBoxLreal.Text = binReader.ReadDouble ( ).ToString ( );
}
else if ( e.notificationHandle == handles [ 5 ] )
{
textBoxReal.Text = binReader.ReadSingle ( ).ToString ( );
}
else if ( e.notificationHandle == handles [ 6 ] )
{
textBoxString.Text = binReader.ReadPlcString ( e.length ).ToString ( );
}
}
}
}