Reading and writing string variables
Requirements
Visual Studio 2008
Target Windows CE Device
TwinCAT ADS WCF 1.1.0.1
Description
Read a string from a plc variable and display it in the visualization or write a string from the visualization to a plc variable.
The TwinCAT.Ads DLL (Compact Framework) is used to read the data from the received byte array, or to write the data into the byte array which will be send to the service, in an easy to handle way.
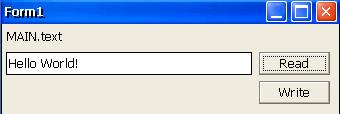
Compact Framework 3.5 (C#) application
using System;
using System.Windows.Forms;
using TcAdsWcf_Sample05.TwinCAT.Ads.Wcf;
using TwinCAT.Ads;
namespace TcAdsWcf_Sample05
{
public partial class Form1 : Form
{
private const string NETID = "10.1.128.80.1.1";
private const int PORT = 801;
private UnsecBasicHttpEndpoint wcfClient;
public Form1 ( )
{
InitializeComponent ( );
// Initialize the WCF client.
wcfClient = new UnsecBasicHttpEndpoint ( );
wcfClient.Url = "http://10.1.128.80:8003/TwinCAT/Ads/Wcf/TcAdsService/UnsecBasicHttp";
}
private void btnRead_Click ( object sender, EventArgs e )
{
// Read the whole plc array into the byte array buffer.
byte[] buffer = wcfClient.Read1 (NETID, PORT, true, "MAIN.text", 30, true);
// Create an AdsStream and an AdsBinaryReader to read the data from the buffer.
AdsStream stream = new AdsStream ( buffer );
AdsBinaryReader binReader = new AdsBinaryReader ( stream );
tbText.Text = binReader.ReadPlcString ( 30 );
}
private void btnWrite_Click ( object sender, EventArgs e )
{
// Create a byte array buffer.
// Length of text +1 because of zero termination.
byte [ ] buffer = new byte [ tbText.Text.Length + 1 ];
// Initialize an AdsStream and an AdsBinaryWriter to write the text to the buffer.
AdsStream stream = new AdsStream ( buffer );
AdsBinaryWriter binWriter = new AdsBinaryWriter ( stream );
binWriter.WritePlcString ( tbText.Text, tbText.Text.Length + 1 );
// Send the buffer.
wcfClient.Write1 ( NETID, PORT, true, "MAIN.text", buffer );
}
}
}