Reading and writing string variables
Requirements
Visual Studio 2010
TwinCAT ADS WCF 1.1.0.1
Description
Read a string from a plc variable and display it in the visualization or write a string from the visualization to a plc variable.
The TwinCAT.Ads DLL is used to read the data from the received byte array, or to write the data into the byte array which will be send to the service, in an easy-to-handle way.
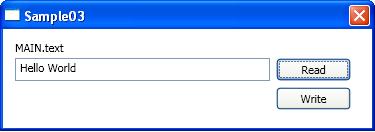
WPF (C#) application
using System.ServiceModel;
using System.Windows;
using TcAdsWcf_Sample03.TwinCAT.Ads.Wcf;
using TwinCAT.Ads;
namespace TcAdsWcf_Sample03
{
public partial class MainWindow : Window
{
// If the netid string is empty, the TwinCAT ADS WCF service will use the TwinCAT Runtime on its host machine.
private const string NETID = "";
private const int PORT = 801;
private TcAdsServiceSimplexClient wcfClient;
public MainWindow ( )
{
InitializeComponent ( );
// Initialize simplex client with BasicHttpBinding.
wcfClient = new TcAdsServiceSimplexClient (
new BasicHttpBinding ( BasicHttpSecurityMode.None ),
new EndpointAddress ( "http://localhost:8003/TwinCAT/Ads/Wcf/TcAdsService/UnsecBasicHttp" ) );
// Open connection to the WCF service.
wcfClient.Open ( );
}
private void BtnRead_Click ( object sender, RoutedEventArgs e )
{
// Read the data from the plc into a byte array buffer.
byte[] buffer = wcfClient.Read1(NETID, PORT, "MAIN.text", 30);
// Initialize an AdsStream and an AdsBinaryWriter to read the data from the buffer.
AdsStream stream = new AdsStream ( buffer );
AdsBinaryReader binReader = new AdsBinaryReader ( stream );
string text = binReader.ReadPlcString ( 30 );
TbText.Text = text;
}
private void BtnWrite_Click ( object sender, RoutedEventArgs e )
{
// Create a byte array buffer.
// Length of text +1 because of zero termination.
byte[] buffer = new byte[ TbText.Text.Length + 1 ];
// Initialize an AdsStream and an AdsBinaryWriter to write the text to the buffer.
AdsStream stream = new AdsStream ( buffer );
AdsBinaryWriter binWriter = new AdsBinaryWriter ( stream );
binWriter.WritePlcString ( TbText.Text, TbText.Text.Length + 1 );
// Send the buffer.
wcfClient.Write1 ( NETID, PORT, "MAIN.text", buffer );
}
}
}