Accessing an array in the PLC
Requirements
Visual Studio 2010
TwinCAT ADS WCF 1.1.0.1
Description
Reading an array of integer variables from the PLC by the use of the TwinCAT ADS WCF service.
The TwinCAT.Ads DLL is used to read the data from the received byte array in an easy to handle way.
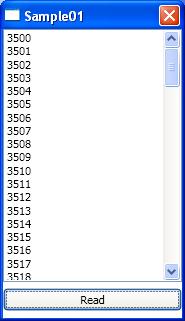
WPF (C#) application
using System.Windows;
using System.ServiceModel;
using TwinCAT.Ads;
using Sample01.TwinCAT.Ads.Wcf;
namespace Sample01
{
public partial class MainWindow : Window
{
// If the netid string is empty, the TwinCAT ADS WCF service will use the TwinCAT Runtime on its host machine.
private const string NETID = "";
private const int PORT = 801;
private TcAdsServiceSimplexClient wcfClient;
public MainWindow ( )
{
InitializeComponent ( );
// Initialize simplex client with BasicHttpBinding.
wcfClient = new TcAdsServiceSimplexClient (
new BasicHttpBinding( BasicHttpSecurityMode.None ),
new EndpointAddress ( "http://localhost:8003/TwinCAT/Ads/Wcf/TcAdsService/UnsecBasicHttp" )
);
// Open connection to the WCF service.
wcfClient.Open ( );
}
private void btnRead_Click ( object sender, RoutedEventArgs e )
{
// Create buffer as byte array which recieves the data.
int buffLen = 100 * 2;
// Read array from plc.
byte[] buffer = wcfClient.Read1(NETID, PORT, "MAIN.PLCVar", buffLen);
// Create AdsStream and AdsBinaryReader for buffer handling.
AdsStream dataStream = new AdsStream ( buffer );
AdsBinaryReader binRead = new AdsBinaryReader ( dataStream );
lbArray.Items.Clear ( );
dataStream.Position = 0;
for ( int i = 0; i < 100; i++ )
{
lbArray.Items.Add ( binRead.ReadInt16( ) );
}
}
}
}