Write string to PLC or read array from PLC
System requirements:
- Delphi 7.0 or higher;
- TwinCAT v2.11 Build 2034 or higher;
Task
A string is to be written to or read from the PLC.
Description
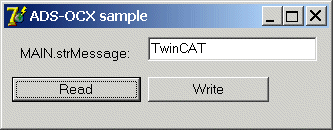
So that a string can be written to or read from the PLC, you need the length of the PLC string. The actual length of a PLC string can be determined using the PLC operator SIZEOF. In the PLC, the strings are terminated with a null and the actual string length is calculated from the defined length plus 1. If no length was specified during the string definition, then the string has an actual length of 81 characters including the terminating null.
PLC program
PROGRAM MAIN
VAR
strColor :STRING(10) :='Blue';
strState :STRING(20) :='STOP';
strMessage :STRING :='TwinCAT ADS-OCX';
END_VAR
strColor has a length of 11 characters;
strState has a length of 21 characters;
strMessage has a length of 81 characters;
Delphi 7 program
unit Unit1;
interface
uses
Windows, Messages, SysUtils, Variants, Classes, Graphics, Controls, Forms,
Dialogs, StdCtrls, OleCtrls, ADSOCXLib_TLB, Grids, ValEdit, ComCtrls;
type
TForm1 = class(TForm)
btnWrite: TButton;
AdsOcx1: TAdsOcx;
Label1: TLabel;
Edit1: TEdit;
btnRead: TButton;
procedure btnReadClick(Sender: TObject);
procedure btnWriteClick(Sender: TObject);
procedure FormCreate(Sender: TObject);
procedure FormDestroy(Sender: TObject);
private
{ Private declarations }
adsResult : Integer;// Ads return code
hVar : Integer;// PLC variable handle
varString : WideString;// PLC variable value
public
{ Public declarations }
end;
var
Form1: TForm1;
implementation
{$R *.dfm}
Reading a string from the PLC
procedure TForm1.btnReadClick(Sender: TObject);
begin
SetLength(varString, 7 );//Realocate string space to a given length// Read string from PLC
adsResult := AdsOcx1.AdsSyncReadStringVarReq( hVar, Length(varString) * 2, varString );
if adsResult = 0 then
edit1.Text := varString
else ShowMessage( Format( 'AdsSyncReadStringVarReq() error:%d', [adsResult] ) );
end;
In the sample above, seven characters of a PLC string were read in Delphi. The dynamic string types have a length of zero immediately after initialization. The Delphi string variable must first be allocated the correct length if the ADS-OCX is to be able to copy the PLC string into the Delphi string variable. In Delphi, a WideString variable requires two bytes for each character. The Length function returns the localized number of characters in the string. However, the Length parameter in the method call requires the byte length, so the length determined with Length function is doubled.
Writing a string into the PLC
procedure TForm1.btnWriteClick(Sender: TObject);
begin
varString := Edit1.Text;
// Write string to the PLC
adsResult := AdsOcx1.AdsSyncWriteStringVarReq( hVar, Length(varString)*2, varString );
if adsResult <> 0 then
ShowMessage( Format( 'AdsSyncWriteStringVarReq() error:%d', [adsResult] ) );
end;
Establish connection to PLC, fetch variable handle
procedure TForm1.FormCreate(Sender: TObject);
begin
// Connection Setup
AdsOcx1.AdsAmsServerNetId := AdsOcx1.AdsAmsServerNetId;
AdsOcx1.AdsAmsServerPort := 801;
// Create variable handle
adsResult := AdsOcx1.AdsCreateVarHandle( 'MAIN.STRMESSAGE', hVar );
if adsResult <> 0 then
ShowMessage( Format( 'AdsCreateVarHandle() error:%d', [adsResult] ) );
end;
Release resources (variable handle)
procedure TForm1.FormDestroy(Sender: TObject);
var adsResult : Integer;
begin
// Delete variable handle
adsResult := AdsOcx1.AdsDeleteVarHandle( hVar );
if AdsResult <> 0 then
ShowMessage( Format( 'AdsDeleteVarHandle() error:%d', [adsResult] ) );
hVar := 0;
end;
Initialization
IsMultiThread := True;// Setting this system variable makes Delphi's memory manager thread-safe
end.
Language / IDE | Unpack sample program |
---|---|
Delphi XE2 | |
Delphi 7 or higher (classic) |