Send/receive messages via the router
Task
ADS devices can send messages to other ADS devices via the TwinCAT Router. They can be received and evaluated there. It is also possible to write messages to the Windows NT/2000/XP Event Logger.
The following Visual Basic program receives messages from the PLC and displays them on the screen. It is also possible for messages to be written to the Windows NT/2000/XP Event Logger from the Visual Basic program.
Description
In order to be able to receive messages, a filter must first be defined using the AdsEnableLogNotification() method. Here the range of port numbers from which ADS device messages are to be received is given. A second parameter additionally states the type of message (error, note or warning). The OR operator can also be used to combine several message types.
Each time a message is sent from an ADS device and the filter conditions are met, the AdsLogNotification() event is called. Via the parameters the source, the type, the time and the message itself can be determined.
If a message is to be sent with the help of the ADS-OCX, the method AdsLogFmtString() is used for this. The first parameter contains the message type (error, note or warning). The message text can contain up to four placeholders for numerical values. This allows, for instance, values that only become known at runtime to be transmitted. All messages that are sent with ADS-OCX are automatically written in the Windows NT/2000/XP Event Logger.
The Windows NT/2000/XP Event Viewer can be called from the Visual Basic program. This allows all the messages that are in the Event Logger to be examined. The Event Logger and the Event Viewer are standard elements in the Windows NT/2000/XP suites. You will find more details in the Windows NT/2000/XP documentation.
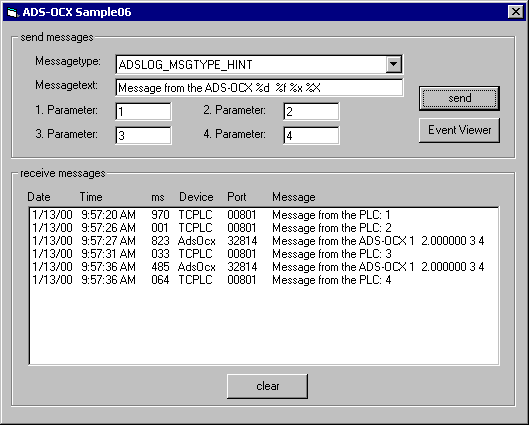
The PLC program sends a note cyclically every 5 seconds. This note is also written into the Windows NT/2000/XP Event Logger. The ADSLOGDINT() PLC function is described in the PLC documentation.
Note: All messages that are sent via the TwinCAT Router can be observed online in the System Manager. This requires Logger output to be activated in the View menu.
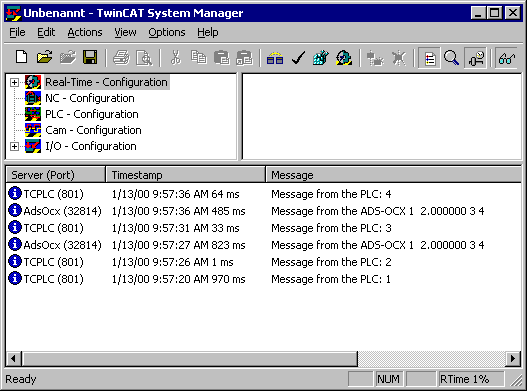
Windows NT/2000/XP contains API functions with which the messages that have been saved in the Event Logger can be read again. Unfortunately, Visual Basic does not (yet) have any components with which the messages saved in the Event Logger can be accessed. On pp. 56 ff of issue 6/98 of the basicopro magazine, published by Steingräberverlag (http://www.basicpro.de), there is an article that illustrates the utilization of the API functions under Visual Basic.
Application
This technique has been found very useful in the development and debugging of applications. A PLC program, for example, or a Visual Basic program can indicate certain internal program states (in the System Manager) and save them (in the Windows NT/2000 Event Logger). For this kind of program tracking it is not necessary to install the development environment (e.g. on a machine computer).
Make sure that not too many messages are transmitted in a short time, otherwise this could affect the overall system.
Hint: If you want to log messages in your program (e.g. malfunctions of a machine), you should use the TwinCAT Event Logger for this purpose. This is significantly more powerful than the Windows NT/2000/XP Event Logger, and is adapted to the requirements of automation technology.
Visual Basic 6 program
Option Explicit
'--- wird beim Starten des Programms aufgerufen ---
Private Sub Form_Load()
cboMessageType.ListIndex = 0
AdsOcx1.EnableErrorHandling = True
'--- Meldungen abfangen ---
Call AdsOcx1.AdsEnableLogNotification(1, 65535, ADSLOG_MSGTYPE_HINT Or ADSLOG_MSGTYPE_ERROR Or ADSLOG_MSGTYPE_WARN)
End Sub
'--- wird beim eintreffen einer Nachricht vom AdsOCX aufgreufen ---
Private Sub AdsOcx1_AdsLogNotification(ByVal dateTime As Date, ByVal nMs As Long, _
ByVal dwMsgCtrl As Long, ByVal nServerPort As Long, _
ByVal szDeviceName As String, ByVal szLogMsg As String)
'--- Meldung anzeigen ---
lstMessages.AddItem Format(DateValue(dateTime), "!@@@@@@@@@@") & _
Format(TimeValue(dateTime), "!@@@@@@@@@@@@@@@") & _
Format(nMs, "000 ") & _
Format(szDeviceName, "!@@@@@@@@@@") & _
Format(nServerPort, "00000 ") & _
szLogMsg
End Sub
'--- Meldung absetzen ---
Private Sub cmdSend_Click()
Dim Para1 As Long
Dim Para2 As Double
Dim Para3 As Integer
Dim Para4 As Integer
'--- Parameter setzen ---
Para1 = CLng(txt1Para.Text)
Para2 = CDbl(txt2Para.Text)
Para3 = CInt(txt3Para.Text)
Para4 = CInt(txt4Para.Text)
'--- Meldung absetzen ---
Call AdsOcx1.AdsLogFmtString(cboMessageType.ItemData(cboMessageType.ListIndex), _
txtMessage.Text, Para1, Para2, Para3, Para4)
End Sub
'--- Ereignisanzeige von Windows NT/2000 anzeigen ---
Private Sub cmdEventViewer_Click()
Call Shell("eventvwr.exe", vbNormalFocus)
End Sub
'--- List löschen ---
Private Sub cmdClearList_Click()
Call lstMessages.Clear
End Sub
PLC program
PROGRAM MAIN
VAR
PLCVarInteger AT %MW0 : INT;
TP_1 : TP;
TOGGEL : BOOL;
AdsLogResult : DINT;
END_VAR
TOGGEL := NOT TOGGEL;
TP_1( IN := TOGGEL, PT := t#5s);
IF (TP_1.Q = 0) THEN
IF (TOGGEL = 0) THEN
PLCVarInteger := PLCVarInteger + 1;
AdsLogResult := ADSLOGDINT(ADSLOG_MSGTYPE_HINT OR ADSLOG_MSGTYPE_LOG , 'Message from the PLC: %d', PLCVarInteger);
END_IF
END_IF
Language / IDE | Unpack sample program |
---|---|
Visual Basic 6 |