Linking into TwinCAT3 (C#)
Creating new project
Start Microsoft Visual Studio and create new project (Windows Forms Application).
Adding reference
In order to select the TwinCAT.Ads class library you must choose the command Add Reference... under the Project menu. You will find the .Net Library per default in following TwinCAT folder:
C:\TwinCAT3\AdsApi\.NET\v2.0.50727
This opens the Add Reference dialog:
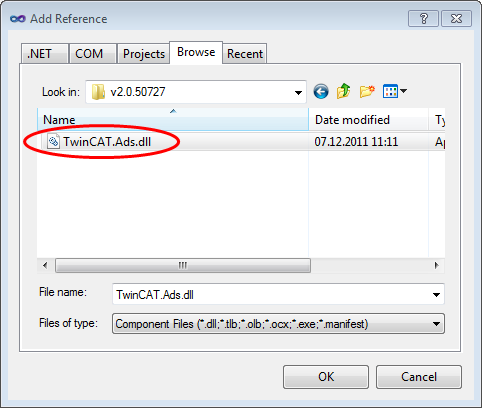
In this dialog you must press the Browse button and select the file TwinCAT.Ads.dll. In the Solution Explorer you can check if the component has been added to the list of references.
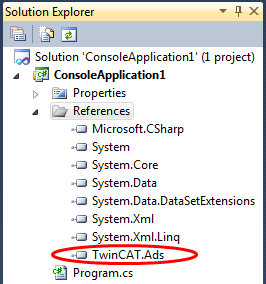
All accessible types (classes, structures ...) belong to the namespace TwinCAT.Ads. Therefore one has to insert the following line at the beginning of the source :
using System.IO, TwinCAT.Ads;
This enables access to the types defined in TwinCAT.Ads without including the name of the namespace. The class TcAdsClient is the core of the TwinCAT.Ads class library and enables the user to communicate with an ads device. To begin with an instance of the class must be created. Then a connection to the ADS device is established by means of the Connect method.
Establish a connection to an ADS device:
TcAdsClient tcAds = new TcAdsClient();
tcAds.Connect("127.0.0.1.1.1",851);
The data is transmitted with the help of the AdsStream class, that inherits form System.IO.MemoryStream. To write data to the stream one can utilize the System.IO.BinaryWriter class and the System.IO.BinaryReader class is necessary to read data from the stream.
Reading a PLC variable:
// creates a stream with a length of 4 byte
AdsStream ds = new AdsStream(4);
BinaryReader br = new BinaryReader(ds);
// reads a DINT from PLC
tcAds.Read(0x4020,0,ds);
ds.Position = 0;
textBox1.Text = br.ReadInt32().ToString();
Writing to a PLC variable:
// creates a stream with a length of 4 byte
AdsStream ds = new AdsStream(4);
BinaryWriter bw = new BinaryWriter(ds);
ds.Position = 0;
bw.Write((int) 100);
// writes a DINT to PLC
tcAds.Write(0x4020,0,ds);
Disconnect a connection to an ADS device:
tcAds.Dispose();
PLC Program:
PROGRAM MAIN
VAR
test AT%MD0 : DINT;
END_VAR
test := test + 1;