Object Function
A function is a POU, which, when executed, returns precisely one data element, and whose call may occur in text-based languages as operator in expressions. The data element may also be an array or a structure.
In the PLC project tree, function POUs have the suffix (FUN). The editor of a function consists of the declaration part and the implementation part.
![]() | All data of a function are temporary and are only valid while the function is executed (stack variables). This means that TwinCAT re-initializes all variables that you have declared in a function each time the function is called. |
![]() | Calls of a function with the same input variable values always return the same output value. Therefore, functions may not use global variables and addresses! |
The top line of the declaration part contains the following declaration:
FUNCTION <function> : <data type>
The input and function variables are declared below.
The output variable of a function is the function name.
![]() | If you declare a local variable in a function as RETAIN, this has no effect. In this case TwinCAT issues a compiler error. |
![]() | In TwinCAT 3, you cannot mix explicit and implicit parameter assignments in function calls. This means that you should either only use explicit or only implicit parameter assignments in function calls. The order of the parameter assignments in function calls is irrelevant. |
Calling a function
In ST you can use function calls as operands in expressions.
In SFC you can use function calls only within step actions or transitions.
Examples:
Function with a declaration part and one line of implementation code:
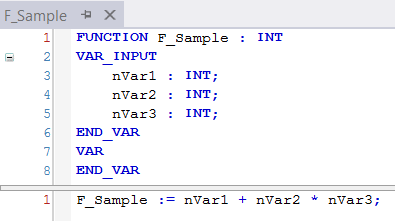
ST:
nRes := F_Sample(5,3,22)
IL:
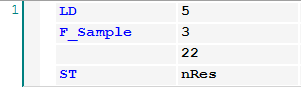
FBD:
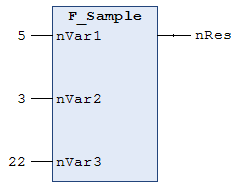
Functions with additional outputs
According to the IEC 61131-3 standard, functions can have additional outputs. You can declare the additional outputs in the function between the keywords VAR_OUTPUT and END_VAR. The function is called based on the following syntax:
<function> (<function output variable1> => <output variable 1>, <function output variable n> => <output variable n>)
Example:
The function F_Fun is defined with two input variables nIn1 and nIn2. The output variable of the function F_Fun is written to the locally declared output variables nLoc1 and nLoc2.
F_Fun(nIn1 := 1, nIn2 := 2, nOut1 => nLoc1, nOut2 => nLoc2);
Access to a single element of a structured return type during method/function/property call
The following implementation can be used to directly access an individual element of the structured data type that is returned by the method/function/property when a method, function or property is called. A structured data type is, for example, a structure or a function block.
- The return type of the method/function/property is defined as "REFERENCE TO <structured type>" (instead of just "<structured type>").
- Note that with such a return type – if, for example, an FB-local instance of the structured data type is to be returned – the reference operator REF= must be used instead of the "normal" assignment operator :=.
The declarations and the sample in this section refer to the call of a property. However, they are equally transferrable to other calls that deliver return values (e.g. methods or functions).
Sample
Declaration of the structure ST_Sample (structured data type):
TYPE ST_Sample :
STRUCT
bVar : BOOL;
nVar : INT;
END_STRUCT
END_TYPE
Declaration of the function block FB_Sample:
FUNCTION_BLOCK FB_Sample
VAR
stLocal : ST_Sample;
END_VAR
Declaration of the property FB_Sample.MyProp with the return type "REFERENCE TO ST_Sample":
PROPERTY MyProp : REFERENCE TO ST_Sample
Implementation of the Get method of the property FB_Sample.MyProp:
MyProp REF= stLocal;
Implementation of the Set method of the property FB_Sample.MyProp:
stLocal := MyProp;
Calling the Get and Set methods in the main program MAIN:
PROGRAM MAIN
VAR
fbSample : FB_Sample;
nSingleGet : INT;
stGet : ST_Sample;
bSet : BOOL;
stSet : ST_Sample;
END_VAR
// Get - single member and complete structure possible
nSingleGet := fbSample.MyProp.nVar;
stGet := fbSample.MyProp;
// Set - only complete structure possible
IF bSet THEN
fbSample.MyProp REF= stSet;
bSet := FALSE;
END_IF
Through the declaration of the return type of the property MyProp as "REFERENCE TO ST_Sample" and through the use of the reference operator REF= in the Get method of this property, a single element of the returned structured data type can be accessed directly on calling the property.
VAR
fbSample : FB_Sample;
nSingleGet : INT;
END_VAR
nSingleGet := fbSample.MyProp.nVar;
If the return type were only to be declared as "ST_Sample", the structure returned by the property would first have to be assigned to a local structure instance. The individual structure elements could then be queried on the basis of the local structure instance.
VAR
fbSample : FB_Sample;
stGet : ST_Sample;
nSingleGet : INT;
END_VAR
stGet := fbSample.MyProp;
nSingleGet := stGet.nVar;