UNION
A UNION is a data structure that usually contains different data types. In a union all components have the same offset, which means they occupy the same memory space.
You declare an union in a DUT object that you create using the command Add > DUT in the context menu of the PLC project tree in the project.
Syntax:
TYPE <union name>:
UNION
<Variable declaration 1>
...
<Variable declaration n>
END_UNION
END_TYPE
Sample 1:
In the following sample declaration of a union, an assignment to uName.fA also affects uName.nB and uName.nC.
Declaration:
TYPE U_Name:
UNION
fA : LREAL;
nB : LINT;
nC : WORD;
END_UNION
END_TYPE
Instantiation:
VAR
uName : U_Name;
END_VAR
Assignment:
uName.fA := 1;
Result:
fA = 1
nB = 16#3FF0000000000000
nC = 0
Sample 2:
In the following sample declaration of a union, an assignment to u2Byte.nUINT also affects u2Byte.a2Byte and u2Byte.aBits.
Declaration of the structure ST_Bits:
TYPE ST_Bits :
STRUCT
bBit1 : BIT;
bBit2 : BIT;
bBit3 : BIT;
bBit4 : BIT;
bBit5 : BIT;
bBit6 : BIT;
bBit7 : BIT;
bBit8 : BIT;
END_STRUCT
END_TYPE
Declaration of the union U_2Byte:
TYPE U_2Byte :
UNION
nUINT : UINT;
a2Byte : ARRAY[1..2] OF BYTE;
aBits : ARRAY[1..2] OF ST_Bits;
END_UNION
END_TYPE
Instantiation of the union:
VAR
u2Byte : U_2Byte;
END_VAR
Assignment 1:
u2Byte.nUINT := 5;
Result 1:
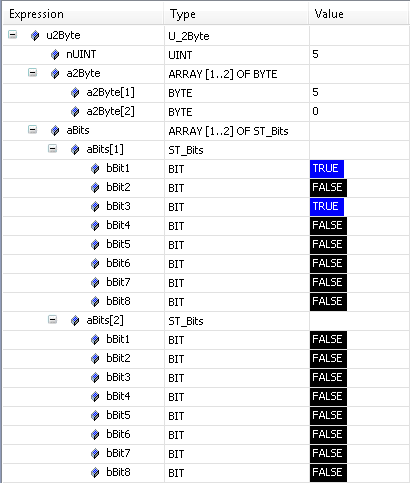
Assignment 2:
u2Byte.nUINT := 255;
Result 2:
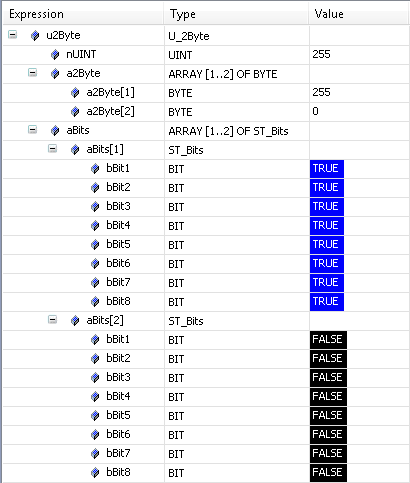
Assignment 3:
u2Byte.nUINT := 256;
Result 3:
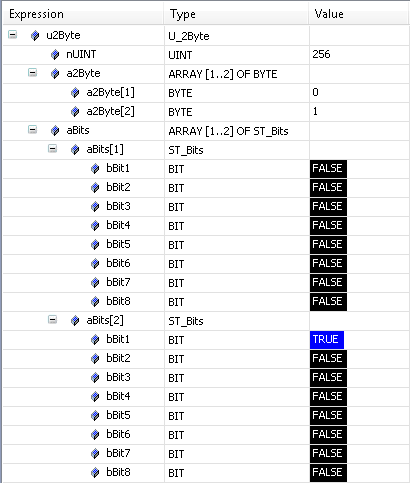
See also: