Structure of program elements
Each new statement/declaration should start on a new line.
The following section explains the structuring of program elements (including POUs, DUTs, GVLs) using text blocks, text sections and text regions.
Text blocks
- Thematically related declarations, assignments or calls with one or no parameter are grouped together in text blocks.
- Calls with more than one parameter, statements (such as IF or CASE) and loops are represented in separate text blocks.
- Each text block starts and ends with a blank line.
- Text blocks can be indented one level within a text region using the tab key, if you want to take advantage of being able to collapse the code below the region comment. If you use indentation, this should be implemented consistently within the POU.
Text sections
- Thematically related text blocks are bundled in text sections.
- Each text section starts and ends with a blank line.
- The first line of a text sections contains comments relating to the following text blocks.
- Text sections can be indented one level within a text region using the tab key, if you want to take advantage of being able to collapse the code below the region comment. If you use indentation, this should be implemented consistently within the POU.
Text regions
- Thematically related text sections are bundled in text regions.
- Each text region starts and ends with a dividing line within a comment.
- The first line below the separator contains comments relating to the following text sections, and therefore the text region. This region comment is on a level with the comment characters above.
- Region comments are not indented. If the text blocks and sections contained in the region are indented, all lines of the text region except for the region comment can thus be expanded or collapsed.
Sample
There are 2 text regions ("Drill settings" and "Conveyor settings") with 2 text sections ("Positions" and "Velocities"). In the text region "Drill settings", the text section "Velocities" consists of 2 text blocks (thematically related associations and an IF statement).
Program code:
//=========================================================
// Drill settings
// Positions
fbDrill.nPositionLower := 100;
fbDrill.nPositionTop := 500;
// Velocities
fbDrill.fVelocityRated := 40.0;
fbDrill.fVelocityMax := 100.0;
IF fbDrill.fVelocityAverage > fbDrill.fVelocityRated THEN
bWarning := TRUE;
sWarning := 'Drill velocity: Average exceeded rated';
END_IF
//=========================================================
// Conveyor settings
// Positions
fbConveyor.nPositionFilling := 50;
fbConveyor.nPositionDrill := 200;
fbConveyor.nPositionDischarge := 300;
// Velocities
fbConveyor.fVelocityRated := 75.5;
//=========================================================
Expanded text regions:
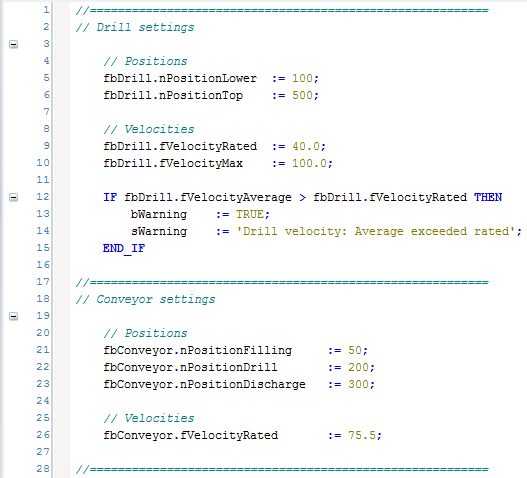
Collapsed text regions:
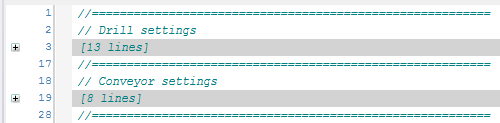
See also:
- Alternative possibility of realizing a folding capability: Region pragma