Creating and handling CANOpen devices
This article explains how to create and handle CANOpen Master and Slave devices via TwinCAT Automation Interface. It consists of following main points:
- Creating and adding a CANOpen Master
- Searching appropriate devices (EL6751, FC510x, etc.) and configuring
- Creating and adding CANOpen Slave
- Searching appropriate devices (EL6751-0010, etc.) and configuring
- Importing DBC files via Automation Interface
Creating and adding a CANOpen Master
To create a CANOpen Master device, open a new or an existing TwinCAT configuration, scan all the devices. Note that these actions can also be performed via Automation Interfaces
Create a system manager object, and navigate to devices.
Code Snippet (C#):
project = solution.Projects.Item(1);
sysman = (ITcSysManager)project.Object;
ITcSmTreeItem io = (ITcSmTreeItem)sysman.LookupTreeItem("TIID");
Code Snippet (Powershell):
$project = $sln.Projects.Items(1)
$sysman = $project.Object
$io = $sysman.LookupTreeItem("TIID")
To add a CANOpen Master, use ITcSmTreeItem:CreateChild method:
Code Snippet (C#):
ITcSmTreeItem5 can_master = (ITcSmTreeItem5)io.CreateChild("Device 2 (EL6751)", 87, "", null);
Code Snippet (Powershell):
$can_master = $io.CreateChild("Device 2 (EL6751)", "87", "", $null)
For other CANOpen master devices, enter the correct ItemSubtype listed here. This will add the new device as shown in the screenshot:
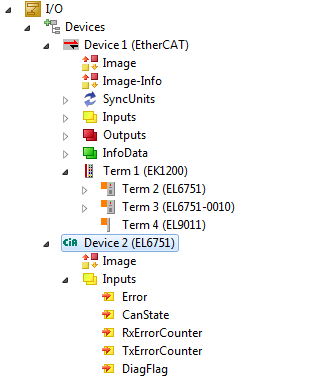
Searching and claiming a CANOpen Master device from the list:
It is necessary to configure the newly added CANOpen master, which is usually done in TwinCAT by pressing the search button and selecting the correct device from the list:
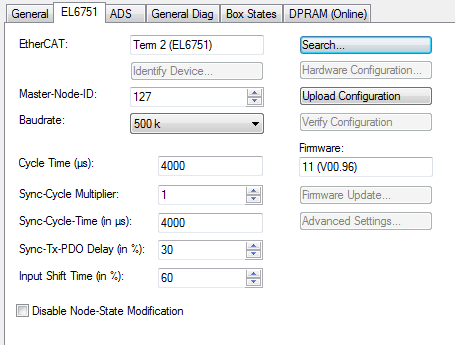
This can be done via Automation Interface:
Code Snippet (C#):
string availableMaster = can_master.ResourceCount;
can_master.ClaimResources(1);
Code Snippet (Powershell):
$availableMaster = $can_master.ResourceCount
$can_master.ClaimResources(1)
The ITcSmTreeItem5:ResourceCount gives out the number of compatible CANOpen master devices and the ITcSmTreeItem5:ClaimResources takes the index of the CANOpen device to be configured as the master.
Creating and adding a CANOpen Slave
A CANOpen slave can be added to the current configuration as shown below:
Code Snippet (C#):
ITcSmTreeItem5 can_slave = (ITcSmTreeItem5)io.CreateChild("Device 3 (EL6751-0010)", "98", null);
Code Snippet (Powershell):
$can_slave = $io.CreateChild("Device 3 (EL6751-0010)", "98", $null)
Searching and claiming a CANOpen slave
Similar to CANOpen Master, a list of CANOpen slaves can be published by following code:
Code Snippet (C#) :
string availableSlaves = can_slave.ResourceCount;
can_slave.Claimresources(1);
Code Snippet (Powershell) :
$availableSlaves = $can_slave.ResourceCount
$can_slave.Claimresources(1)
The last line of code, claims the EL6751-0010 as the CANOpen slave, similar to the dialog box that appears in TwinCAT user interface.
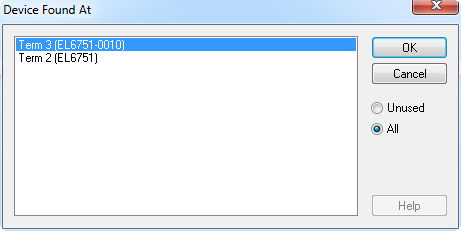
Importing DBC files via Automation Interface
Required Version: TwinCAT 3.1 Build 4018 or above
TwinCAT offers DBC file to be imported via dialog box as seen in the screenshot. On right clicking a CANOpen Master e.g. EL6751 and selecting “Import dbc-file”, a dialog box prompts user to browse the DBC file. Upon clicking okay, a CANOpen configuration is loaded automatically.
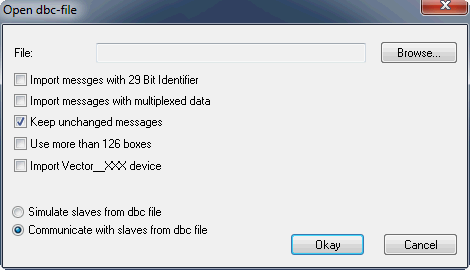
Automation Interface also supports DBC file import. To do this, navigate to the CANOpen Master tree item and export the Xml file via ITcSmTreeItem:ProduceXml().
In the Xml file, add the path to the DBC file to be imported along with the additional properties that are part of the dialog box shown above.
<DeviceDef>
<AmsPort>28673</AmsPort>
<AmsNetId>172.17.251.109.2.1</AmsNetId>
<AddressInfo>
<Ecat>
<EtherCATDeviceId>0</EtherCATDeviceId>
</Ecat>
</AddressInfo>
<MaxBoxes>126</MaxBoxes>
<ScanBoxes>false</ScanBoxes>
<CanOpenMaster>
<ImportDbcFile>
<FileName>c:\dbc_file_folder\dbc_file_to_be_imported.dbc</FileName>
<ImportExtendedMessages>false</ImportExtendedMessages>
<ImportMultiplexedDataMessages>false</ImportMultiplexedDataMessages>
<KeepUnchangedMessages>true</KeepUnchangedMessages>
<ExtBoxesSupport>false</ExtBoxesSupport>
<VectorXXXSupport>false</VectorXXXSupport>
<CommunicateWithSlavesFromDbcFile>true</CommunicateWithSlavesFromDbcFile>
</ImportDbcFile>
</CanOpenMaster>
<Fcxxxx>
<CalculateEquiTimes>0</CalculateEquiTimes>
<NodeId>127</NodeId>
<Baudrate>500 k</Baudrate>
<DisableNodeStateModification>false</DisableNodeStateModification>
</Fcxxxx>
</DeviceDef>
Import the modified Xml file back into the TwinCAT configuration via ITcSmTreeImte:ConsumeXml()
. The configuration will now be loaded below the CANOpen Master.