Attribute 'TcCallAfterOutputUpdate'
The attribute pragma TcCallAfterOutputUpdate
causes the IO update to take place before the PLC cycle and not after the PLC program as is set by default.
This function can be used for projects with strongly fluctuating cycle times. In projects with strongly fluctuating cycle times, the outputs, since they are written after the PLC cycle, are sometimes written earlier (short PLC cycle time) and sometimes later (long PLC cycle time). These fluctuations cause jitter in the outputs. The disadvantage is that the attribute cannot react quite as quickly and a cycle is always lost. You have to decide whether you want to react quickly to an input (default setting) or whether you prefer to have a deterministic behavior of the outputs (setting of the attribute).
Syntax: {attribute 'TcCallAfterOutputUpdate'}
Insertion location: This attribute must be added to all program POUs, which are to be called after the output update.
Sample:
To illustrate the behavior, you need a digital output terminal such as an EL2008 and an oscilloscope.
Write a small PLC program and link the variable bOut
with a digital output:
bOut:=not bOut;
The PLC program is very simple and does not cause any fluctuations. The pulse is displayed on the oscilloscope as follows:
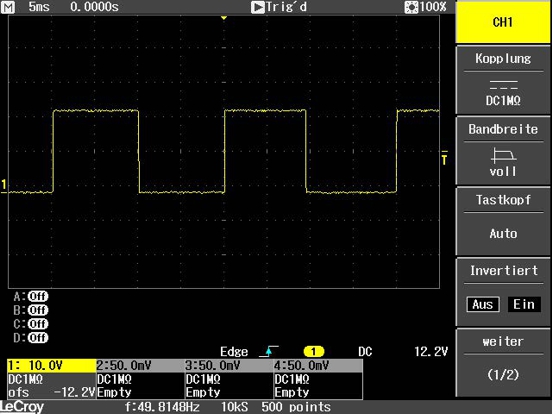
Now extend the PLC program with a For loop to create a program load. The mathematical function used does not matter and is intended only to generate a load:
bOut:=not bOut;
IF bOut THEN
For loop:=1 to 2000 do
lrTest:=SIN(INT_TO_LREAL(loop)*3.14);
END_FOR
END_IF
Whenever the output is set to TRUE, the loop is run through and a load is generated. As a result, more time is needed to run the PLC and the output is written later than usual. During the next cycle, the output is set back to FALSE, the loop is not run through and the output is set to FALSE faster, because the PLC program is finished faster without a For loop. The result is that the pulse is very much shorter.
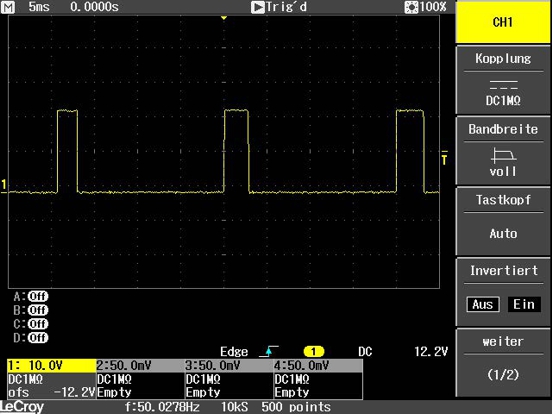
If the For loop is called upon FALSE instead of TRUE, the result is inverted.
bOut:=not bOut;
IF not bOut THEN
For loop:=1 to 2000 do
lrTest:=SIN(INT_TO_LREAL(loop)*3.14);
END_FOR
END_IF
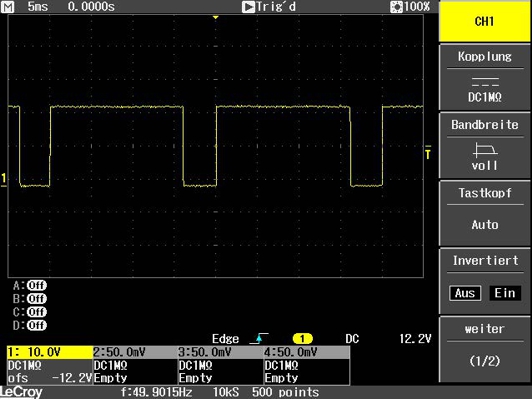
With the attribute pragma TcCallAfterOutputUpdate
, the pulse is constant and is independent of how long the For loop takes or whether it is called. The whole thing only works if the PLC task is not exceeded. Therefore, when reproducing the sample, pay attention to the exceed counters of the task.
Detecting a PLC program with different runtimes
The PLC program must be supplemented in order to detect PLC programs with different runtimes. Different runtimes are not recognizable in the online view, since an average value is always formed over several cycles. Therefore, outliers can only be detected if they lie above the task time. If the outliers are still within the task time, they are not easily visible.
For this we then use the system variable: PlcTaskSystemInfo
VAR
bOut : BOOL;
PlcTaskSystemInfo : PlcTaskSystemInfo;
udiValue : ARRAY[0..19] of UDINT;
Cnt : INT;
END_VAR
Program:
bOut:=not bOut;
IF bOut THEN
For loop:=1 to 2000 do
lrTest:=SIN(INT_TO_LREAL(loop)*3.14);
END_FOR
END_IF
PlcTaskSystemInfo:=_TaskInfo[1];
udiValue[Cnt]:= PlcTaskSystemInfo.LastExecTime;
cnt:=cnt+1;
IF Cnt >19 THEN
Cnt:=0;
END_IF
With this program extension you can see that the PLC program with a For loop requires 7.7 ms and without a For loop 1.1 ms. The specification is 100 ns per digit.
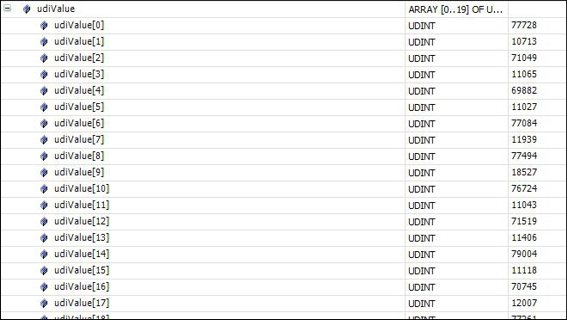
The measurement coincides with the displays on the oscilloscope, on which it can be seen that a pulse is sometimes 6.5 ms longer or 6.5 ms shorter. You can measure the processing time of the For loop (Measuring processing time in the PLC program). The result of this measurement will coincide with the observed values through the program extension, with a certain inaccuracy and jitter.