Structure of the CAN interface
The CAN interface looks different depending on the options selected. An 11-bit identifier interface is structured differently to a 29-bit identifier interface. In addition, the interface may contain the Transaction Number as well as the Time Stamp. When using structures the target system must be considered and which alignment it supports. Corresponding attributes are usable under TwinCAT 3 {attribute 'pack_mode':= '0'}.
The interface consists of the communication with the interface and up to 32 CAN messages. The inputs can only be read if the outputs are written.
The interface is addressed as follows:
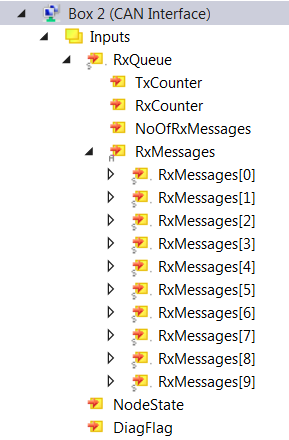
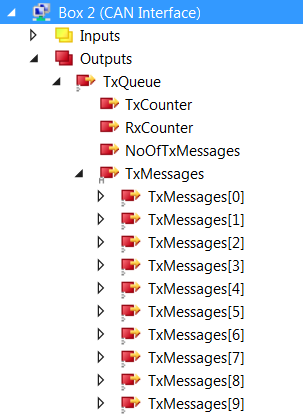
Outputs.TxCounter is set to +1 if data are to be transmitted. NoOfTxMessages also indicates how many messages are to be transmitted from the buffer. The RxCounter indicates whether there are new data in the buffer. NoOfRxMessages indicates how many new data are in the buffer.
If you have fetched the data, then set Outputs.RxCounter:=Inputs.RxCounter. The CAN interface then knows that it can fill the buffer again. All data must always be read out, because the CAN interface fills all message structures again when necessary.
Sample code for transmission
if (Outputs.TxCounter = Inputs.TxCounter) // check if the interface is ready
and NumberOfMessagesToSend >0 then // and messages are to send
for i:=0 to NumberOfMessagesToSend-1 do // LOOP for copying the CAN message to the
// interface
Outputs.TxMessage[i] := MessageToSend[i]; // copy
End_for
Outputs.NoOfTxMessages := NumberOfMessagesToSend; // number of CAN messages you are going to send
Outputs.TxCounter := Inputs.TxCounter + 1; // inc. shows the CAN interface that new data
// is available and to send this data
end_if
Sample code for reading
if Outputs.RxCounter <> Inputs.RxCounter then // check if new data is in the buffer
for i := 0 to (Inputs.NoOfRxMessages-1) do // start the LOOP and check how much data
// is in the buffer
MessageReceived[i] := Inputs.RxMessage [i]; // copy the CAN message
End_for
Outputs.RxCounter := Inputs.RxCounter; // set equal: the CAN interface then knows,
// that you have copied the CAN data
end_if
Message structure when using the 11-bit identifier
The message structure when using the 11-bit identifier consists of the COB ID [2 bytes] and the 8 bytes of data. The COB ID has the following structure:
- Bit 0-3: Length of the data (0…8)
- Bit 4: RTR
- Bit 5-15: 11-bit identifier
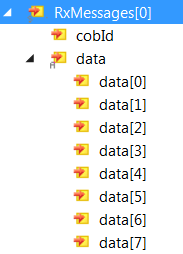
Since COB ID, length and RTR bit are coded in one word in the 11-bit identifier, the following example is helpful for decoding the data from the word. Select a structure here in which to store the decoded data.
IF RXCounter_Out <> RXCounter_In THEN
FOR I := 0 TO (NoOfTxMessages-1) DO
stCANInterfaceMessageValue[i].Lengh:=WORD_TO_BYTE(stCANInterfaceMessage[i].CobID) AND 16#0F;
stCANInterfaceMessageValue[i].RTR:=stCANInterfaceMessage[i].CobID.4;
stCANInterfaceMessageValue[i].CobID :=ROR(stCANInterfaceMessage[i].CobID,5) AND 16#07FF;
stCANInterfaceMessageValue[i].Data := stCANInterfaceMessage[i].Data;
CASE stCANInterfaceMessageValue[i].CobID OF
16#318: COB318:=COB318+1;
16#718: COB718:=COB718+1;
16#1CD: COB1CD:=COB1CD+1;
memcpy(ADR(TempValue),ADR(stCANInterfaceMessage[i].Data[6]),2);
16#1ED: COB1ED:=COB1ED+1;
ELSE
COBALLOther:=COBAllOther+1;
END_CASE
End_for
RXCounter_Out:=RXCounter_In;
END_IF
Message structure when using the 29-bit identifier
The message structure when using the 29-bit identifier consists of the length [2 bytes] of the COB ID [4 bytes] and the 8 bytes of data.
Lenght: Length of the data (0…8)
The COB ID has the following structure:
- Bit 0-28: 29-bit identifier
- Bit 30: RTR
- Bit 31:
- 0: 11-bit identifier,
- 1: 29-bit identifier
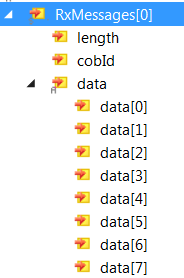