UDP sample: .NET Peer-to-Peer Kommunikation
Dieses Beispiel demonstriert, wie Sie einen .NET Kommunikationspartner für die SPS-Beispiele Peer-to-Peer device A oder B realisieren können.
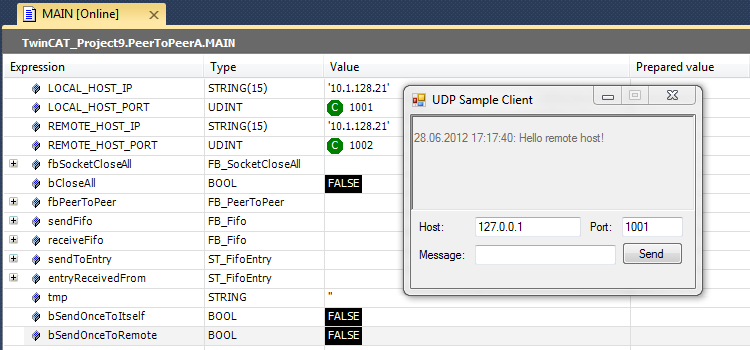
Wie das Beispiel funktioniert
Dieses Beispiel nutzt die .NET Bibliotheken System.Net und System.Net.Sockets, um durch die Klasse UdpClient einen UDP-Client zu realisieren. Parallel zum Empfangen eingehender UDP-Pakete durch einen Background-Thread, kann auch ein String an ein remote-Gerät übermittelt werden, indem dessen IP-Adresse und Portnummer eingegeben und der Button "Send" angeklickt wird.
DIe folgende Anleitung basiert auf dem folgenden Setup:
- Das SPS-Projekt Peer-to-Peer device A läuft auf einem Computer mit der IP-Adresse 10.1.128.21
- Die .NET Anwendung läuft auf einem Computer mit der IP-Adresse 10.1.128.30
Einrichtung des SPS-Projekts
Dieses .NET Beispiel läuft im Zusammenspiel mit den SPS-Beispielen Peer-to-Peer device A oder B. Wenn Sie die Anwendung auf einem anderen Computer als die SPS-Laufzeit ausführen, müssen Sie die IP-Adressen beider Geräte anhand Ihrer tatsächlichen Umgebung entsprechend in den Beispielprojekten anpassen. Die folgende Tabelle geht von oben erwähnten Beispiel-Setup aus:
Einstellung | Typ | Beschreibung |
---|---|---|
LOCAL_HOST_IP | Globale Konstante | 10.1.128.21 (IP-Adresse des Computers mit der SPS-Laufzeit) |
LOCAL_HOST_PORT | Globale Konstante | 1001 (Standard-Wert) |
REMOTE_HOST_IP | Globale Konstante | 10.1.128.30 (IP-Adresse des Computers mit der .NET Anwendung) |
REMOTE_HOST_PORT | Globale Konstante | 1002 (Standard-Wert) |
bSendOnceToRemote | Globale Variable | Wenn auf TRUE gesetzt, wird ein UDP-Paket an die IP-Adresse REMOTE_HOST_IP gesendet |
Einrichtung der .NET Anwendung
Einstellung | Typ | Beschreibung |
---|---|---|
DEFAULTIP | Globale Konstante | 10.1.128.21 (Wird in dem Textfeld "Host" als Standardwert eingetragen.) |
DEFAULTDESTPORT | Globale Konstante | 1001 (Wird in dem Textfeld "Port" als Standardwert eingetragen.) |
DEFAULTOWNPORT | Globale Konstante | 1002 (Entspricht dem Wert der globalen Konstante REMOTE_HOST_PORT im SPS-Projekt, siehe oben.) |
DEFAULTSOURCEPORT | Globale Konstante | Wird als Quell-Port benutzt, über den das UDP-Paket verschickt wird. Dieser kann auf der Standardeinstellung belassen werden. |
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
using System.Net;
using System.Net.Sockets;
using System.Threading;
namespace TcpIpServer_SampleClientUdp
{
publicpartialclassForm1 : Form
{
/* ########################################################################################## * Constants * ########################################################################################## */privateconststring DEFAULTIP = "127.0.0.1";
privateconstint DEFAULTDESTPORT = 1001; // Used as destination portprivateconstint DEFAULTOWNPORT = 1002; // Used for binding when listening for UDP messagesprivateconstint DEFAULTSOURCEPORT = 11000; // Only used as source port when sending UDP messages/* ########################################################################################## * Global variables * ########################################################################################## */privatestaticUdpClient _udpClient;
privatestaticIPEndPoint _ipAddress; // Contains IP address as entered in text fieldprivatestaticThread _rcvThread; // Background thread used to listen for incoming UDP packetspublic Form1()
{
InitializeComponent();
}
/* ########################################################################################## * Event handler method called when button "Send" is pressed * ########################################################################################## */privatevoid cmd_send_Click(object sender, EventArgs e)
{
byte[] sendBuffer = null;
/* ########################################################################################## * Preparing UdpClient, connecting to UDP server and sending content of text field * ########################################################################################## */try
{
_ipAddress = newIPEndPoint(IPAddress.Parse(txt_host.Text), Convert.ToInt32(txt_port.Text));
_udpClient = newUdpClient(DEFAULTSOURCEPORT);
_udpClient.Connect(_ipAddress);
sendBuffer = Encoding.ASCII.GetBytes(txt_send.Text);
_udpClient.Send(sendBuffer, sendBuffer.Length);
_udpClient.Close();
}
catch (Exception ex)
{
MessageBox.Show("An unknown error occured: " + ex);
}
}
/* ########################################################################################## * Event handler method called when application starts * ########################################################################################## */privatevoid Form1_Load(object sender, EventArgs e)
{
txt_host.Text = DEFAULTIP;
txt_port.Text = DEFAULTDESTPORT.ToString();
rtb_rcv.Enabled = false;
/* ########################################################################################## * Creating background thread which synchronously listens for incoming UDP packets * ########################################################################################## */
_rcvThread = newThread(rcvThreadMethod);
_rcvThread.Start();
}
/* ########################################################################################## * Delegate, so that background thread may write into text field on GUI * ########################################################################################## */publicdelegatevoidrcvThreadCallback(string text);
/* ########################################################################################## * Method called by background thread * ########################################################################################## */privatevoid rcvThreadMethod()
{
/* ########################################################################################## * Listen on any available local IP address and specified port (DEFAULTOWNPORT) * ########################################################################################## */byte[] rcvBuffer = null;
IPEndPoint ipEndPoint = newIPEndPoint(IPAddress.Any, DEFAULTOWNPORT);;
UdpClient udpClient = newUdpClient(ipEndPoint);
/* ########################################################################################## * Continously start a synchronous listen for incoming UDP packets. If a packet has arrived, * write its content to receive buffer and then into the text field. After that, start circle * again. * ########################################################################################## */while (true)
{
rcvBuffer = udpClient.Receive(ref ipEndPoint); // synchronous call
rtb_rcv.Invoke(newrcvThreadCallback(this.AppendText), newobject[] { "\n" + DateTime.Now.ToString() + ": " + Encoding.ASCII.GetString(rcvBuffer) });
}
}
/* ########################################################################################## * Helper method for delegate * ########################################################################################## */privatevoid AppendText(string text)
{
rtb_rcv.AppendText(text);
}
/* ########################################################################################## * Stop background thread when application closes * ########################################################################################## */privatevoid Form1_FormClosed(object sender, FormClosedEventArgs e)
{
_rcvThread.Abort();
}
}
}