Accessing an array in the PLC
Requirements
Visual Studio 2008
Target Windows CE Device
TwinCAT ADS WCF 1.1.0.1
Description
Reading an array of integer variables from the PLC using the TwinCAT ADS WCF service.
The TwinCAT.Ads DLL (Compat Framework) is used to read the data from the received byte array in an easy-to-handle way.
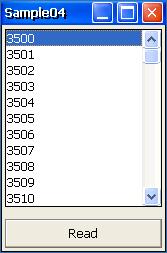
Compact Framework 3.5 (C#) application
using System;
using System.Windows.Forms;
using TcAdsWcf_Sample04.TwinCAT.Ads.Wcf;
using TwinCAT.Ads;
namespace TcAdsWcf_Sample04
{
public partial class Form1 : Form
{
private const string NETID = "10.1.128.80.1.1";
private const int PORT = 801;
private UnsecBasicHttpEndpoint wcfClient;
public Form1 ( )
{
InitializeComponent ( );
// Initialize the WCF client.
wcfClient = new UnsecBasicHttpEndpoint ( );
wcfClient.Url = "http://10.1.128.80:8003/TwinCAT/Ads/Wcf/TcAdsService/UnsecBasicHttp";
}
private void btnRead_Click ( object sender, EventArgs e )
{
// Read the whole plc array into the byte array buffer.
int buffLen = 100 * 2;
byte[] buffer = wcfClient.Read1(NETID, PORT, true, "MAIN.PLCVar", buffLen, true );
// Create an AdsStream and an AdsBinaryReader to read the data from the buffer.
AdsStream stream = new AdsStream ( buffer );
AdsBinaryReader binReader = new AdsBinaryReader ( stream );
stream.Position = 0;
lbArray.Items.Clear ( );
for ( int i = 0; i < 100; i++ )
{
lbArray.Items.Add ( binReader.ReadInt16 ( ).ToString ( ) );
}
}
}
}