Event driven reading with Visual Basic
Task
There are 7 global variables in the PLC. Each of these PLC variables is of a different data type. The values of the variables should be read in the most effective manner, and the value with its timestamp is to be displayed on a form.
Description
In the form's MainForm_Load event, a connection to each of the PLC variables is created with the TcAdsClient.AddDeviceNotification() method. The handle for this connection is stored in a array.
The parameter TransMode specifies the type of data exchange. AdsTransMode.OnChange has been selected here. This means that the value of the PLC variable is only transmitted if its value within the PLC has changed (see the AdsTransMode data type). The parameter cycleTime indicates that the PLC is to check whether the corresponding variable has changed every 100 ms. MaxDelay allows to collect notification for a specified interval. If the maxDelay elapse, all notifications will be send at once.
When the PLC variable changes, the TcAdsClient.AdsNotification() event is called. The parameter e of the event handling method is of the type AdsNotificationEventArgs and contains the time stamp, the handle, the value and the control in which the value is to be displayed. The connections are released again in the MainForm_FormClosing event by means of the TcAdsClient.DeleteDeviceNotification() method. It is essential that you do this, since every connection established by TcAdsClient.AddDeviceNotification() uses resources.
You should also choose appropriate values for the cycle time, since too many write / read operations load the system so heavily that the user interface becomes much slower.
Advice: Don't use time intensive executions in callbacks (OnNotification()).
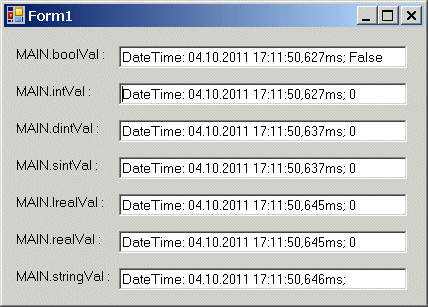
Visual Basic (for .NET framework) program
Imports TwinCAT.Ads
Imports System.IO
Public Class Form1
Inherits System.Windows.Forms.Form
#Region " Windows Form Designer generated code "
...
#End Region
' Handle events (1): without withevents declaration
Private tcClient As TcAdsClient
' Handle events (2):You can also declare tcClient as:
' Private WithEvents tcClient As TcAdsClient
Private hConnect() As Integer
Private dataStream As AdsStream
Private binRead As BinaryReader
Private Sub Form1_Load(ByVal sender As Object, ByVal e As System.EventArgs) Handles MyBase.Load
Dim dataStream As New AdsStream(31)
' Set encoding to ASCII to be able to read strings
binRead = New BinaryReader(dataStream, System.Text.Encoding.ASCII)
' Create instance of TcAdsClient class
tcClient = New TcAdsClient()
' Establish connection to local port 801
tcClient.Connect(801)
ReDim hConnect(7)
Try
hConnect(0) = tcClient.AddDeviceNotification("MAIN.boolVal", dataStream, 0, 1, _
AdsTransMode.OnChange, 100, 0, tbBool)
hConnect(1) = tcClient.AddDeviceNotification("MAIN.intVal", dataStream, 1, 2, _
AdsTransMode.OnChange, 100, 0, tbInt)
hConnect(2) = tcClient.AddDeviceNotification("MAIN.dintVal", dataStream, 3, 4, _
AdsTransMode.OnChange, 100, 0, tbDint)
hConnect(3) = tcClient.AddDeviceNotification("MAIN.sintVal", dataStream, 7, 1, _
AdsTransMode.OnChange, 100, 0, tbSint)
hConnect(4) = tcClient.AddDeviceNotification("MAIN.lrealVal", dataStream, 8, 8, _
AdsTransMode.OnChange, 100, 0, tbLreal)
hConnect(5) = tcClient.AddDeviceNotification("MAIN.realVal", dataStream, 16, 4, _
AdsTransMode.OnChange, 100, 0, tbReal)
hConnect(6) = tcClient.AddDeviceNotification("MAIN.stringVal", dataStream, 20, 11, _
AdsTransMode.OnChange, 100, 0, tbString)
' Handle events (1):
AddHandler tcClient.AdsNotification, AddressOf OnNotification
Catch err As Exception
MessageBox.Show(err.Message)
End Try
End Sub
Private Sub Form1_Closing(ByVal sender As Object, ByVal e As System.ComponentModel.CancelEventArgs) Handles MyBase.Closing
Try
tcClient.Dispose()
Catch err As Exception
MessageBox.Show(err.Message)
End Try
End Sub
' Handle events (2):
' Private Sub OnNotification(ByVal sender As Object, ByVal e As AdsSyncNotificationEventArgs) Handles tcClient.AdsSyncNotification
' Handle events (1):
Private Sub OnNotification(ByVal sender As Object, ByVal e As AdsNotificationEventArgs)
Dim time As New DateTime()
time = DateTime.FromFileTime(e.TimeStamp)
e.DataStream.Position = e.Offset
Dim strValue As String = ""
If (e.NotificationHandle = hConnect(0)) Then
strValue = binRead.ReadBoolean().ToString()
ElseIf (e.NotificationHandle = hConnect(1)) Then
strValue = binRead.ReadInt16().ToString()
ElseIf (e.NotificationHandle = hConnect(2)) Then
strValue = binRead.ReadInt32().ToString()
ElseIf (e.NotificationHandle = hConnect(3)) Then
strValue = binRead.ReadByte().ToString()
ElseIf (e.NotificationHandle = hConnect(4)) Then
strValue = binRead.ReadDouble().ToString()
ElseIf (e.NotificationHandle = hConnect(5)) Then
strValue = binRead.ReadSingle().ToString()
ElseIf (e.NotificationHandle = hConnect(6)) Then
strValue = New String(binRead.ReadChars(11))
End If
Dim tb As New TextBox()
tb = CType(e.UserData, TextBox)
tb.Text = String.Format("DateTime: {0},{1}ms; {2}", time, time.Millisecond, strValue)
End Sub
End Class
PLC program
PROGRAM MAIN
VAR
boolVal : BOOL;
intVal : INT;
dintVal : DINT;
sintVal : SINT;
lrealVal : LREAL;
realVal : REAL;
stringVal : STRING(10);
END_VAR
;