Transmitting a structure to the PLC with Visual Basic
Task
A structure is to be written into the PLC by the .NET application. The elements in the structure have different data types.
Description
The structure we want to write into the PLC:
TYPE PLCStruct
STRUCT
intVal : INT; (*Offset 0*)
dintVal : DINT; (*Offset 2*)
byteVal : SINT; (*Offset 6*)
lrealVal : LREAL;(*Offset 7*)
realVal : REAL; (*Offset 15 --> Total size 19 Bytes*)
END_STRUCT
END_TYPE
The AdsStream object is initialized with a size of 19 bytes in the write event method. This is equivalent to the size of the data type PLCStruct in the SPS. A BinaryWriter is used to write the data from the textboxes to the stream. After setting the stream position to 0 the individual fields are written to the stream. Finally the total stream is written into the SPS with the help of TcAdsClient.Write.
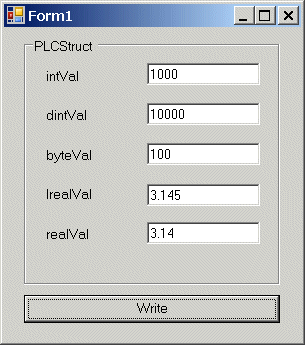
Visual Basic (for .NET framework) program
Imports TwinCAT.Ads
Imports System.IO
Public Class Form1
Inherits System.Windows.Forms.Form
#Region " Windows Form Designer generated code "
...
#End Region
Dim hVar As Integer
Dim tcClient As TcAdsClient
Private Sub Form1_Load(ByVal sender As Object, ByVal e As System.EventArgs) Handles MyBase.Load
' Create instance of TcAdsClient
tcClient = New TcAdsClient()
' Establish connection to local system port 801
tcClient.Connect(801)
Try
hVar = tcClient.CreateVariableHandle("MAIN.PLCVar")
Catch err As Exception
MessageBox.Show(err.Message)
End Try
End Sub
Private Sub Form1_Closing(ByVal sender As Object, ByVal e As System.ComponentModel.CancelEventArgs) Handles MyBase.Closing
' Release resources
Try
tcClient.DeleteVariableHandle(hVar)
Catch err As Exception
MessageBox.Show(err.Message)
End Try
tcClient.Dispose()
End Sub
Private Sub btnWrite_Click(ByVal sender As Object, ByVal e As System.EventArgs) Handles btnWrite.Click
Dim dataStream As New AdsStream(19)
Dim binWrite As New BinaryWriter(dataStream)
dataStream.Position = 0
Try
binWrite.Write(Short.Parse(tbInt.Text))
binWrite.Write(Integer.Parse(tbDint.Text))
binWrite.Write(Byte.Parse(tbByte.Text))
binWrite.Write(Double.Parse(tbLReal.Text))
binWrite.Write(Single.Parse(tbReal.Text))
tcClient.Write(hVar, dataStream)
Catch err As Exception
MessageBox.Show(err.Message)
End Try
End Sub
End Class
PLC program
TYPE PLCStruct :
STRUCT
intVal : INT;
dintVal : DINT;
sintVal : SINT;
lrealVal : LREAL;
realVal : REAL;
END_STRUCT
END_TYPE
PROGRAM MAIN
VAR
PLCVar:PLCStruct;
END_VAR