Accessing an array in the PLC with Visual Basic
Task
The PLC contains an array that is to be read by the .Net application using a read command.
Description
The PLC contains an array of 100 elements of type integer (2 bytes). The array in the PLC is to be filled with the values from 3500 to 3599.
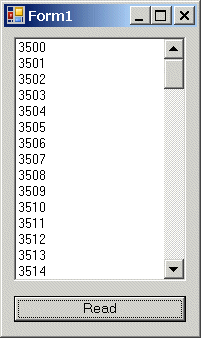
Visual Basic (for .NET framework) program
Imports TwinCAT.Ads
Imports System.IO
Public Class Form1
Inherits System.Windows.Forms.Form
#Region " Windows Form Designer generated code "
...
#End Region
Dim hVar As Integer
Dim tcClient As TcAdsClient
Private Sub Form1_Load(ByVal sender As Object, ByVal e As System.EventArgs) Handles MyBase.Load
tcClient = New TcAdsClient()
tcClient.Connect(801)
Try
hVar = tcClient.CreateVariableHandle("MAIN.PLCVar")
Catch err As Exception
MessageBox.Show(err.Message)
End Try
End Sub
Private Sub Form1_Closing(ByVal sender As Object, ByVal e As System.ComponentModel.CancelEventArgs) Handles MyBase.Closing
'Release resources
Try
tcClient.DeleteVariableHandle(hVar)
Catch err As Exception
MessageBox.Show(err.Message)
End Try
tcClient.Dispose()
End Sub
Private Sub btnRead_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles btnRead.Click
Try
Dim dataStream As New AdsStream(100 * 2)
Dim binRead As New BinaryReader(dataStream)
'Read complete array
tcClient.Read(hVar, dataStream)
lbArray.Items.Clear()
dataStream.Position = 0
Dim i As Integer
For i = 0 To 99
lbArray.Items.Add(binRead.ReadInt16().ToString())
Next
Catch err As Exception
MessageBox.Show(err.Message)
End Try
End Sub
End Class
PLC program
PROGRAM MAIN
VAR
PLCVar : ARRAY [0..99] OF INT;
Index: BYTE;
END_VAR
FOR Index := 0 TO 99 DO
PLCVar[Index] := 3500 + INDEX;
END_FOR