Using Templates
The following article describes how to use templates instead of configuring every single setting separately via Automation Interface. The usage of templates provides many advantages: they are easier to maintain, easier to replace with new templates, and they provide great possibilities when working with multiple teams on one TwinCAT project. This document describes the usage of templates and covers the following topics:
- The general idea behind templates and its different levels
- Working with I/O templates
- Working with Motion templates (axes)
- Working with PLC templates
The general idea behind templates and its different levels
The idea behind the usage of templates is to simplify Automation Interface code and the Automation Interface application itself. Most users are not aware that, in a TwinCAT configuration, templates can exist on multiple levels. These include:
- Templates on “configuration level”
- Templates on configuration level may exist as several TwinCAT configurations (*.sln or *.tszip file). Each configuration may include different content and can be opened and activated by Automation Interface code.
- Templates on “(PLC) project level”
- Templates on (PLC) project level may exist as several TwinCAT PLC Projects, either as an unpacked folder structure (*.plcproj file) or as a container (*.tpzip) file. These PLC Projects can then be imported on demand via Automation Interface code.
- Templates on “tree item level”
- Templates on tree item level may exist as so-called TwinCAT export files (*.xti). These files can be imported on demand via Automation Interface code and contain all settings of a tree item, e.g. settings that have been made to an EtherCAT Master I/O device.
As the description from above might suggest, all different template levels have one thing in common: the templates exist as files in the file system. It is best practice that, at the beginning of implementing an own Automation Interface application, one clearly defines what kind of “template pool” is being used. A template pool describes a repository, where all template files (which might also be a mix of different template levels) are being stored. This could simply be the local (or remote) file system, or might also be a source control system (e.g. Team Foundation Server), from which template files are automatically retrieved, checked out and put together to a new TwinCAT configuration. For your convenience and to make the first steps easier for you, we have prepared a separate documentation article that demonstrates how to use the Visual Studio Object Model (DTE) to connect to a Team Foundation Server (TFS) and execute these operations via program code. However, to get more information about using TFS via Automation Interface code, we heavily suggest to read the corresponding articles from the Microsoft Developer Network (MSDN).
The following topics describe how each template level can be used in Automation Interface for a different TwinCAT area (I/O, PLC, …).
Working with configuration templates
Templates on configuration level provide the most basic way of using templates. In this scenario, a template pool holds two or more pre-configured TwinCAT configurations and provides them as TwinCAT Solution files (*.sln or *.tszip). These files can be opened via Automation Interface by using the Visual Studio method AddFromTemplate().
Code Snippet (C#):
project = solution.AddFromTemplate(@"C:\TwinCAT Project.tszip", destination, projectName);
Code Snippet (Powershell):
$project = $solution.AddFromTemplate(@"C:\TwinCAT Project.tszip", $destination, $projectName)
OR
Code Snippet (C#):
project = solution.Open(@"C:\TwinCAT Project 1.sln");
Code Snippet (Powershell):
$project = $solution.Open(@"C:\TwinCAT Project 1.sln")
Working with I/O templates
When working with I/O devices, users often experience the same tasks on I/O devices over and over again. That means, making the same settings to I/O devices with each TwinCAT configuration. TwinCAT provides a mechanism that stores all these settings in a special file format, the so-called XTI file (*.xti). This file format can be used by Automation Interface code to be imported to a new configuration by using the method ImportChild() which is defined in the interface ITcSmTreeItem.
Code Snippet (C#):
ITcSmTreeItem io = systemManager.LookupTreeItem("TIID");
ITcSmTreeItem newIo = io.ImportChild(@"C:\IoTemplate.xti", "", true, "SomeName");
Code Snippet (Powershell):
$io = $systemManager.LookupTreeItem("TIID")
$newIo = $io.ImportChild(@"C:\IoTemplate.xti", "", true, "SomeName")
It is important to note that, if you export an I/O device from within TwinCAT XAE, that all sub devices are automatically exported and included in the export file as well. The following screenshot shows how to export I/O devices into an export file.
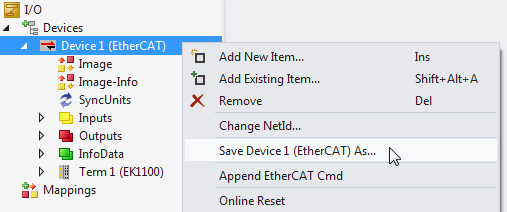
Please note that you could also use the Automation Interface to export an I/O device into an XTI file. For this, the method ExportChild() from the ITcSmTreeItem interface is provided.
Working with Motion templates (axes)
The usage of Motion axes templates is very similar to I/O devices. Axes can also be exported into an XTI-file, either via TwinCAT XAE or via Automation Interface code by using ExportChild().
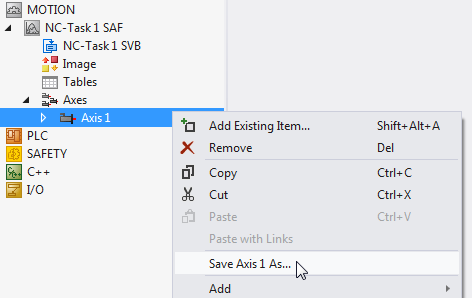
By using ImportChild(), these export files can later be imported again.
Code Snippet (C#):
ITcSmTreeItem motionAxes = systemManager.LookupTreeItem("TINC^NC-Task^Axes");
motionAxes.ImportChild(@"C:\AxisTemplates.xti", "", true, "Axis 1");
Code Snippet (Powershell):
$motionAxes = $systemManager.LookupTreeItem("TINC^NC-Task^Axes")
$motionAxes.ImportChild(@"C:\AxisTemplates.xti", "", true, "Axis 1")
Working with PLC templates
PLC templates are available in two units: You can either use the complete PLC projects as a whole or each POU individually as a templates. To integrate the former into an existing TwinCAT project, simply use the CreateChild() method of the ITcSmTreeItem interface.
Code Snippet (C#):
ITcSmTreeItem plc = systemManager.LookupTreeItem("TIPC");
plc.CreateChild("NewPlcProject", 0, null, pathToTpzipOrTcProjFile);
Code Snippet (Powershell):
$plc = $systemManager.LookupTreeItem("TIPC")
$plc.CreateChild("NewPlcProject", 0, $null, $pathToTpzipOrTcProjFile)
More options to import existing PLC projects can be found in the best practice article about “Accessing, creating and handling PLC projects”.
The next granularity level of importing template files for the PLC is to import existing POUs, like function blocks, structs, enums, global variable lists, etc. One of the reasons a developer may choose individual POUs as templates, is that it is easier to build a pool of existing functionalities and encapsulate them in separate POUs, e.g. different function blocks covering different sorting algorithms. Upon project creation, the developer simply chooses which functionality he needs in his TwinCAT project and accesses the template tool to retrieve the corresponding POU and import it to his PLC project.
Code Snippet (C#):
ITcSmTreeItem plcProject = systemManager.LookupTreeItem(“TIPC^Name^Name Project”);
plcProject.CreateChild(“NameOfPou”, 58, null, pathToPouFile);
plcProject.CreateChild(null, 58, null, stringArrayWithPathsToPouFiles);
Code Snippet (Powershell):
$plcProject = $systemManager.LookupTreeItem("TIPC^Name^Name Project")
$plcProject.CreateChild("NameOfPou", 58, $null, $pathToPouFile)
$plcProject.CreateChild($null, 58, $null, $stringArrayWithPathsToPouFiles)
Note | |
Importing one or more templates A POU template file may not only be a .TcPou file but also the corresponding files for DUTs and/or GVLs. The example above demonstrates two common ways to import POU template files. The first is to import a single file, the second to import multiple files at once by storing the file paths to a string array and using this string array as the vInfo parameter of CreateChild(). |