Creating and handling TwinCAT Measurement projects
The TwinCAT Automation Interface provides methods and properties to create and access TwinCAT Measurement projects. The following chapter explains how to solve some basic tasks with that kind of TwinCAT project and includes information about the following topics:
- Requirements
- Creating a TwinCAT Measurement project
- Creating a TwinCAT Scope configuration
- Creating, accessing and handling charts
- Creating, accessing and handling axes
- Creating, accessing and handling channels
- Starting and stopping records
- For more information about TwinCAT Measurement, please visit the corresponding webpage in our Information System.
Requirements
Integrating TwinCAT Measurement projects via Automation Interface is available since TwinCAT 3.1 Build 4013.
As a prerequisite to use the Scope AI functionalities, a reference to the following COM interfaces is required:
- TwinCAT Measurement Automation Interface (registered as COM library)
- TwinCAT Scope2 Communications (.NET assembly: C:\Windows\Microsoft.NET\assembly\GAC_MSIL\TwinCAT.Scope2.Communications\v4.0_3.1.3121.0__180016cd49e5e8c3\)
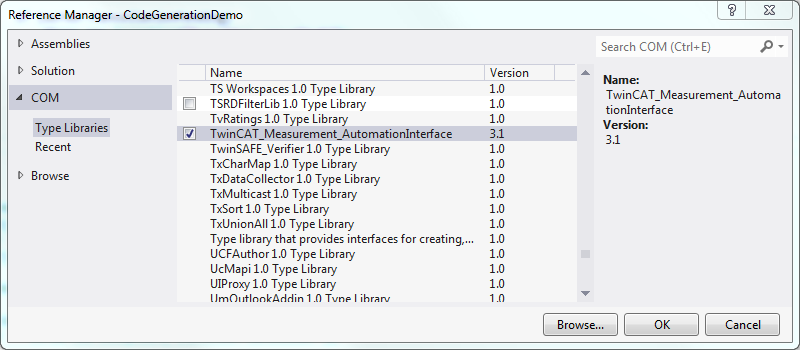
Creating a TwinCAT Measurement project
TwinCAT Measurement is a global "container" which can host one or more measurement projects, e.g. a TwinCAT Scope configuration. Similar to a regular TwinCAT configuration, each project is first-of-all described by a template-file. This template file is used when adding a new project to the solution, which can be achieved by calling the AddFromTemplate() method from the Visual Studio DTE object. Please note that this procuedure is the same when adding a regular TwinCAT project. The following code snippet assumes that you have already acquired a DTE instance and created a Visual Studio solution, as demonstrated in our article about Accessing TwinCAT configurations. A reference to the DTE instance is stored in the object "dte".
Code Snippet (C#):
EnvDTE.Project scopeProject = dte.Solution.AddFromTemplate(template, destination, name);
[template]: The default template files are stored under C:\TwinCAT\Functions\TE130X-Scope-View\Templates\Projects\ and have the file type "tcmproj".
[destination]: Path where the new configuration should be stored on hard disk.
[name]: Name for the new configuration, as displayed in TwinCAT XAE.
Creating a TwinCAT Scope configuration
A TwinCAT Scope project stands for a recording configuration. This means that all elements inserted in that project are subject to the same recording settings. You can add a Scope project via Automation Interface by specifying the corresponding "TwinCAT Scope Project" template when adding a project using the AddFromTemplate() method, as described above.
Creating, accessing and handling charts
Several charts can exist in parallel in a Scope configuration. To add charts to an existing Scope project, simply use the CreateChild() method from the IMeasurementScope interface. The following code snippet assumes that a TwinCAT Measurement Project has already been created and a reference to this project is stored in the object "scopeProject".
Code Snippet (C#):
((IMeasurementScope)scopeProject.ProjectItems.Item(1).Object).ShowControl();
EnvDTE.ProjectItem newChart;
((IMeasurementScope)scopeProject.ProjectItems.Item(1).Object).CreateChild(out newChart);
IMeasurementScope newChartObj = (IMeasurementScope)newChart.Object;
newChartObj.ChangeName("NC Chart");
The object "newChartObj" now stores a reference to the newly added chart. At this point, please keep in mind that we still need the original object "newChart" for later purposes, e.g. to set chart properties.
In TwinCAT XAE, chart properties are set via the Visual Studio properties window. The object "newChart" gives access to these properties via its collection "Properties". The following code snippet iterates through all properties of a chart and sets the property "StackedYAxis" to "true".
Code Snippet (C#):
foreach (EnvDTE.Property prop in newChart.Properties)
{
If (prop.Name = "StackedYAxis")
prop.Value = true;
}
Creating, accessing and handling axes
The following code snippet demonstrates how to add axes.
Code Snippet (C#):
EnvDTE.ProjectItem newAxis;
newChartObj.CreateChild(out newAxis);
IMeasurementScope newAxisObj = (IMeasurementScope)newAxis.Object;
newAxisObj.ChangeName(“Axis 1”);
Creating, accessing and handling channels
A Scope channel scribes a connection to a runtime symbol, e.g. a PLC variable. To add channels via Automation Interface, you can use the CreateChild() method of the IMeasurementScope interface.
Code Snippet (C#):
EnvDTE.ProjectItem newChannel;
newAxisObj.CreateChild(out newChannel);
IMeasurementScope newChannelObj = (IMeasurementScope)newChannel.Object;
newChannelObj.ChangeName("Signals.Rectangle");
The object "newChannelObj" now stores a reference to the newly added channel. At this point, please keep in mind that we still need the original object "newChannel" for later purposes, e.g. to set channel properties.
In TwinCAT XAE, channel properties are set via the Visual Studio properties window. The object "newChannel" gives access to these properties via its collection "Properties". The following code snippet iterates through all properties of a channel and sets multiple properties.
Code Snippet (C#):
foreach (EnvDTE.Property prop in newChannel.Properties)
{
switch (prop.Name)
{
case "TargetPorts":
prop.Value = "851";
break;
case "Symbolbased":
prop.Value = true;
break;
case "SymbolDataType":
prop.Value = TwinCAT.Scope2.Communications.Scope2DataType.BIT;
break;
case "SymbolName":
prop.Value = "MAIN.bSignal";
break;
case "SampleState":
prop.Value = 1;
break;
case "LineWidth":
prop.Value = 3;
break;
}
}
As you can see, the Scope2DataType enum defines several data types that are supported when configuring a channel. At creation time of this document, this enum is defined as follows:
Code Snippet (C#):
public enum Scope2DataType
{
VOID = 0,
BIT = 1,
INT16 = 2,
INT32 = 3,
INT64 = 4,
INT8 = 5,
REAL32 = 6,
REAL64 = 7,
UINT16 = 8,
UINT32 = 9,
UINT64 = 10,
UINT8 = 11,
BIT_ARRAY_8 = 12,
}
Please use a DLL explorer (e.g. Visual Studio) for a more recent and up-to-date list.
Starting and stopping records
To start/stop a configured Scope record, the corresponding methods from the IMeasurementScope interface can be used:
Code Snippet (C#):
((IMeasurementScope)scopeProject.ProjectItems.Item(1).Object).StartRecord()
((IMeasurementScope)scopeProject.ProjectItems.Item(1).Object).StopRecord();